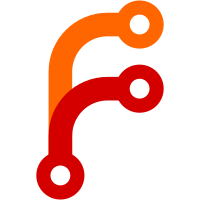
Previously, calling `.right()` on a `Gfx::Rect` would return the last column's coordinate still inside the rectangle, or `left + width - 1`. This is called 'endpoint inclusive' and does not make a lot of sense for `Gfx::Rect<float>` where a rectangle of width 5 at position (0, 0) would return 4 as its right side. This same problem exists for `.bottom()`. This changes `Gfx::Rect` to be endpoint exclusive, which gives us the nice property that `width = right - left` and `height = bottom - top`. It enables us to treat `Gfx::Rect<int>` and `Gfx::Rect<float>` exactly the same. All users of `Gfx::Rect` have been updated accordingly.
48 lines
1.5 KiB
C++
48 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2021, Mustafa Quraish <mustafa@serenityos.org>
|
|
* Copyright (c) 2023, the SerenityOS developers.
|
|
* Copyright (c) 2023, Sam Atkins <atkinssj@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "ClassicSkin.h"
|
|
|
|
namespace Snake {
|
|
|
|
ClassicSkin::ClassicSkin(Color color)
|
|
: m_skin_color(color)
|
|
{
|
|
}
|
|
|
|
void ClassicSkin::draw_tile_at(Gfx::Painter& painter, Gfx::IntRect const& rect)
|
|
{
|
|
painter.fill_rect(rect, m_skin_color.darkened(0.77));
|
|
|
|
Gfx::IntRect left_side(rect.x(), rect.y(), 2, rect.height());
|
|
Gfx::IntRect top_side(rect.x(), rect.y(), rect.width(), 2);
|
|
Gfx::IntRect right_side(rect.right() - 2, rect.y(), 2, rect.height());
|
|
Gfx::IntRect bottom_side(rect.x(), rect.bottom() - 2, rect.width(), 2);
|
|
auto top_left_color = m_skin_color.lightened(0.88);
|
|
auto bottom_right_color = m_skin_color.darkened(0.55);
|
|
painter.fill_rect(left_side, top_left_color);
|
|
painter.fill_rect(right_side, bottom_right_color);
|
|
painter.fill_rect(top_side, top_left_color);
|
|
painter.fill_rect(bottom_side, bottom_right_color);
|
|
}
|
|
|
|
void ClassicSkin::draw_head(Gfx::Painter& painter, Gfx::IntRect const& head, Direction)
|
|
{
|
|
painter.fill_rect(head, m_skin_color);
|
|
}
|
|
void ClassicSkin::draw_body(Gfx::Painter& painter, Gfx::IntRect const& rect, Direction, Direction)
|
|
{
|
|
draw_tile_at(painter, rect);
|
|
}
|
|
void ClassicSkin::draw_tail(Gfx::Painter& painter, Gfx::IntRect const& tail, Direction)
|
|
{
|
|
draw_tile_at(painter, tail);
|
|
}
|
|
|
|
}
|