Publication point
This commit is contained in:
parent
6799dfe35e
commit
4e82174c6d
3 changed files with 186 additions and 0 deletions
|
@ -16,3 +16,8 @@
|
|||
|
||||
By committing source code, data or other material to the Shopimail project you are assigning all copyright and/ or similar rights to AVERWAY LTD
|
||||
in consideration of its agreement to license the source, code, data or other material under GPL v.3.0.
|
||||
|
||||
## Screenshots
|
||||
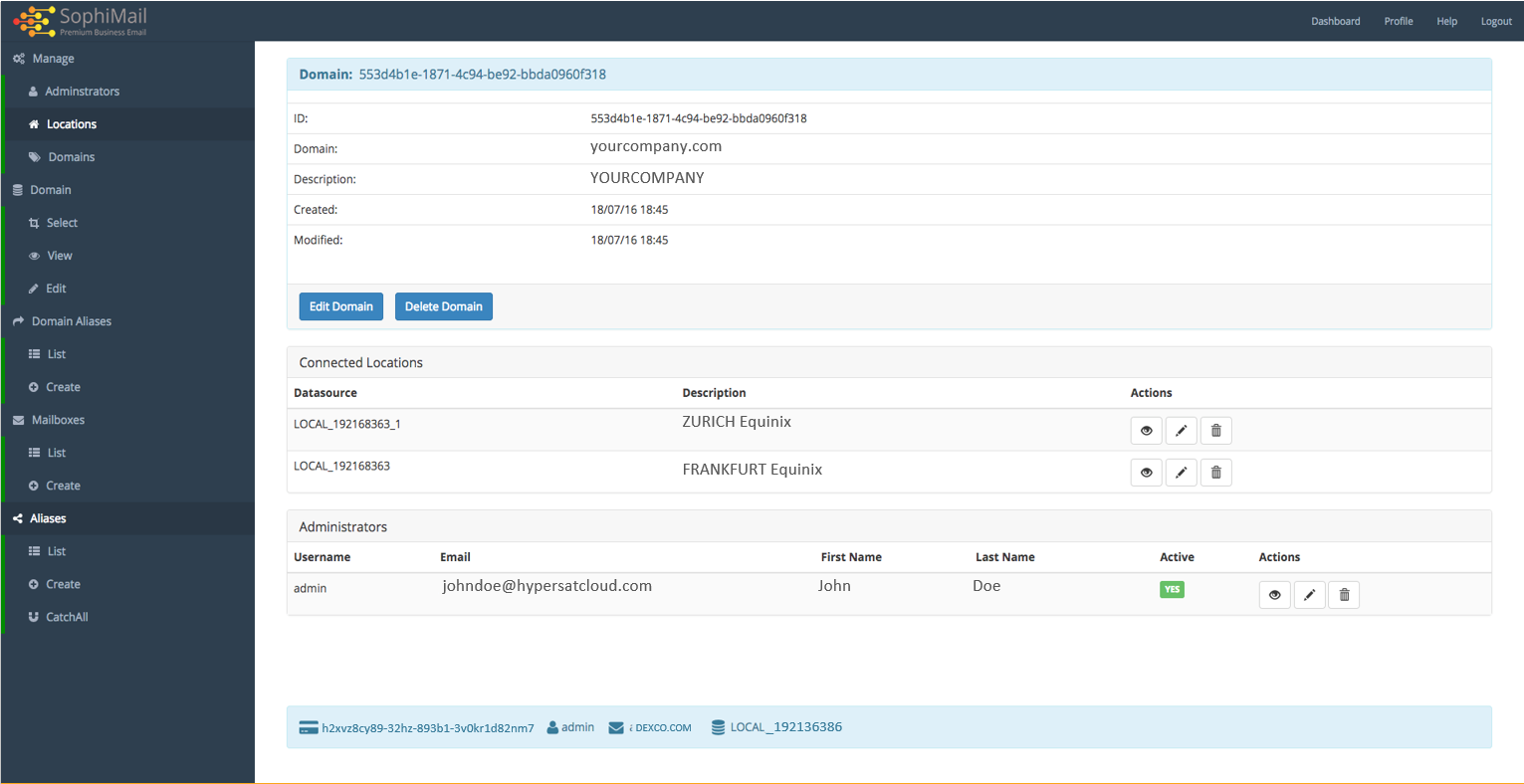
|
||||
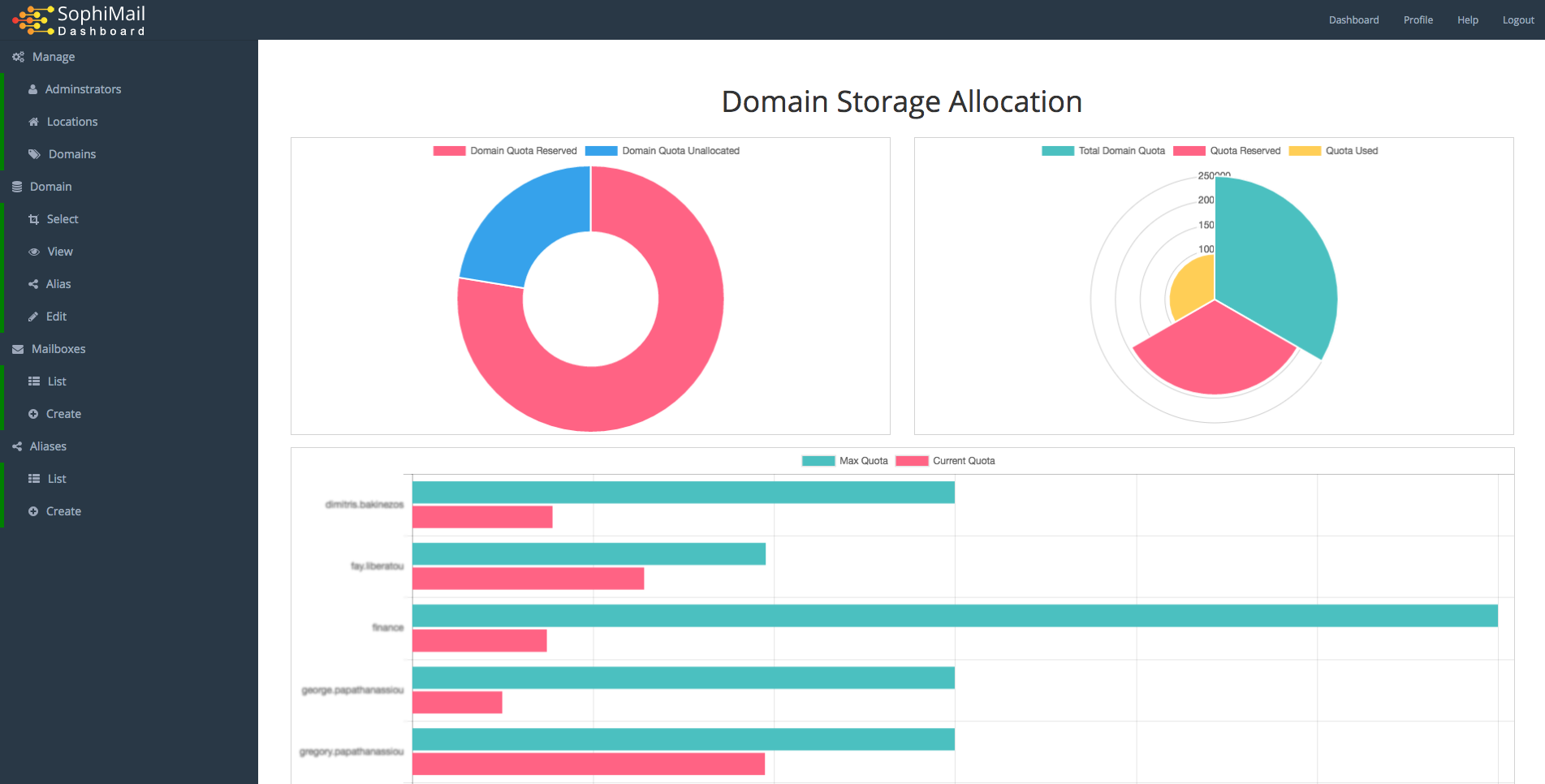
|
||||
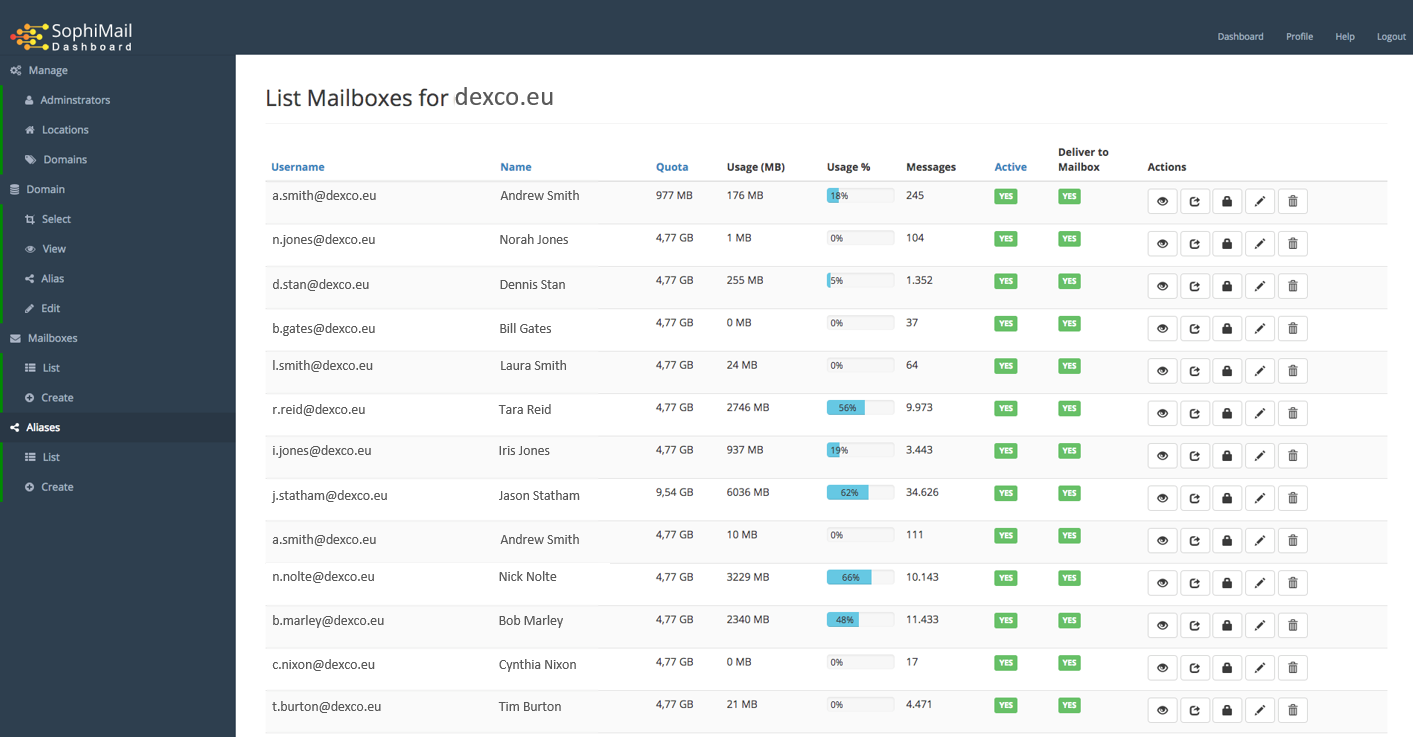
|
||||
|
|
98
src/Controller/AppController.php
Executable file
98
src/Controller/AppController.php
Executable file
|
@ -0,0 +1,98 @@
|
|||
<?php
|
||||
/**
|
||||
*
|
||||
* Copyright (c) 2017 AVERWAY LTD
|
||||
*
|
||||
* LICENSE:
|
||||
*
|
||||
* This file is part of SophiMail Dashboard (http://www.sophimail.com).
|
||||
*
|
||||
* SophiMail Dashboard is free software: you can redistribute it and/or modify it under the terms of the GNU General Public
|
||||
* License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any
|
||||
* later version with exceptions for vendors and plugins.
|
||||
*
|
||||
* See the LICENSE file for a full license statement.
|
||||
*
|
||||
* SophiMail Dashboard is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied
|
||||
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
|
||||
* details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License along with SophiMail Dashboard. If not, see
|
||||
* <http://www.gnu.org/licenses/>.
|
||||
*
|
||||
*
|
||||
* @author AVERWAY LTD <support@sophimail.com>
|
||||
* @license GNU/GPLv3 or later; https://www.gnu.org/licenses/gpl.html
|
||||
* @copyright 2017 AVERWAY LTD
|
||||
*
|
||||
* SophiMail is a registered trademark of AVERWAY LTD
|
||||
*
|
||||
*/
|
||||
namespace App\Controller;
|
||||
|
||||
use Cake\Controller\Controller;
|
||||
use Cake\Event\Event;
|
||||
use Cake\Core\Configure;
|
||||
|
||||
/**
|
||||
* Application Controller
|
||||
*
|
||||
* Add your application-wide methods in the class below, your controllers
|
||||
* will inherit them.
|
||||
*
|
||||
* @link http://book.cakephp.org/3.0/en/controllers.html#the-app-controller
|
||||
*/
|
||||
class AppController extends Controller
|
||||
{
|
||||
|
||||
/**
|
||||
* Initialization hook method.
|
||||
*
|
||||
* Use this method to add common initialization code like loading components.
|
||||
*
|
||||
* e.g. `$this->loadComponent('Security');`
|
||||
*
|
||||
* @return void
|
||||
*/
|
||||
public function initialize()
|
||||
{
|
||||
parent::initialize();
|
||||
|
||||
$this->loadComponent('RequestHandler');
|
||||
$this->loadComponent('Flash');
|
||||
$this->loadComponent('CakeDC/Users.UsersAuth');
|
||||
$this->loadComponent('Global');
|
||||
|
||||
}
|
||||
|
||||
/*
|
||||
public function beforeFilter(Event $event)
|
||||
{
|
||||
$this->Security->config('unlockedActions', ['register']);
|
||||
}
|
||||
*/
|
||||
|
||||
/**
|
||||
* Before render callback.
|
||||
*
|
||||
* @param \Cake\Event\Event $event The beforeRender event.
|
||||
* @return void
|
||||
*/
|
||||
public function beforeRender(Event $event)
|
||||
{
|
||||
if (!array_key_exists('_serialize', $this->viewVars) &&
|
||||
in_array($this->response->type(), ['application/json', 'application/xml'])
|
||||
) {
|
||||
$this->set('_serialize', true);
|
||||
}
|
||||
}
|
||||
|
||||
public function beforeFilter(Event $event){
|
||||
if ($this->Global->_UserDomainDatasourceExists()) {
|
||||
Configure::write('_domain', $this->Global->_getDomain());
|
||||
Configure::write('_datasource', $this->Global->_getDatasource());
|
||||
} else {
|
||||
$this->Global->_deleteParams();
|
||||
}
|
||||
}
|
||||
}
|
83
src/Controller/Component/GlobalComponent.php
Executable file
83
src/Controller/Component/GlobalComponent.php
Executable file
|
@ -0,0 +1,83 @@
|
|||
<?php
|
||||
/**
|
||||
*
|
||||
* Copyright (c) 2017 AVERWAY LTD
|
||||
*
|
||||
* LICENSE:
|
||||
*
|
||||
* This file is part of SophiMail Dashboard (http://www.sophimail.com).
|
||||
*
|
||||
* SophiMail Dashboard is free software: you can redistribute it and/or modify it under the terms of the GNU General Public
|
||||
* License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any
|
||||
* later version with exceptions for vendors and plugins.
|
||||
*
|
||||
* See the LICENSE file for a full license statement.
|
||||
*
|
||||
* SophiMail Dashboard is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied
|
||||
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
|
||||
* details.
|
||||
*
|
||||
* You should have received a copy of the GNU General Public License along with SophiMail Dashboard. If not, see
|
||||
* <http://www.gnu.org/licenses/>.
|
||||
*
|
||||
*
|
||||
* @author AVERWAY LTD <support@sophimail.com>
|
||||
* @license GNU/GPLv3 or later; https://www.gnu.org/licenses/gpl.html
|
||||
* @copyright 2017 AVERWAY LTD
|
||||
*
|
||||
* SophiMail is a registered trademark of AVERWAY LTD
|
||||
*
|
||||
*/
|
||||
namespace App\Controller\Component;
|
||||
|
||||
use Cake\Controller\Component;
|
||||
use Cake\ORM\TableRegistry;
|
||||
use Cake\Core\Configure;
|
||||
|
||||
class GlobalComponent extends Component
|
||||
{
|
||||
|
||||
public function _deleteParams() {
|
||||
$this->request->session()->delete('Auth.User.datasource');
|
||||
$this->request->session()->delete('Auth.User.domain');
|
||||
Configure::delete('_domain');
|
||||
Configure::delete('_datasource');
|
||||
}
|
||||
|
||||
|
||||
public function _getDomain() {
|
||||
if ($this->_UserDomainExists()) {
|
||||
$results = TableRegistry::get('Shards')->get($this->request->session()->read('Auth.User.domain'));
|
||||
return $results['domain'];
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
|
||||
public function _getDatasource() {
|
||||
if ($this->_DomainDatasourceExists()) {
|
||||
$results = TableRegistry::get('Accounts')->get($this->request->session()->read('Auth.User.datasource'));
|
||||
return $results['datasource'];
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
|
||||
public function _UserDomainExists() {
|
||||
if (($this->request->session()->read('Auth.User.id')) && ($this->request->session()->read('Auth.User.domain')))
|
||||
return TableRegistry::get('UsersShards')->exists(['user_id' => $this->request->session()->read('Auth.User.id'), 'shard_id' => $this->request->session()->read('Auth.User.domain')]);
|
||||
}
|
||||
|
||||
|
||||
public function _DomainDatasourceExists() {
|
||||
if (($this->request->session()->read('Auth.User.datasource')) && ($this->request->session()->read('Auth.User.domain')))
|
||||
return TableRegistry::get('ShardsAccounts')->exists(['account_id' => $this->request->session()->read('Auth.User.datasource'), 'shard_id' => $this->request->session()->read('Auth.User.domain')]);
|
||||
}
|
||||
|
||||
|
||||
public function _UserDomainDatasourceExists() {
|
||||
return (($this->_UserDomainExists()) && ($this->_DomainDatasourceExists()));
|
||||
}
|
||||
}
|
Loading…
Reference in a new issue