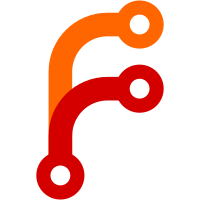
The goal here is to allow Cell::initialize to return a ThrowCompletion, to handle OOM for example. Cell.h will then need to include Completion.h which must include Value.h. This currently can't happen because Value.h includes BigInt.h, which in turn includes Cell.h. So we would have an include cycle. This removes BigInt.h from Value.h, as it is forward-declarable (it is only referred to with a reference or pointer). Then the Value overload for Cell::Visitor::visit is moved to Cell.h, and missing BigInt.h includes as peppered as needed.
46 lines
1.6 KiB
C++
46 lines
1.6 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2020-2021, Linus Groh <linusg@serenityos.org>
|
|
* Copyright (c) 2020-2022, Idan Horowitz <idan.horowitz@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibJS/Runtime/BigInt.h>
|
|
#include <LibJS/Runtime/PrimitiveString.h>
|
|
#include <LibJS/Runtime/Value.h>
|
|
|
|
namespace JS {
|
|
struct ValueTraits : public Traits<Value> {
|
|
static unsigned hash(Value value)
|
|
{
|
|
VERIFY(!value.is_empty());
|
|
if (value.is_string()) {
|
|
// FIXME: Propagate this error.
|
|
return value.as_string().deprecated_string().release_value().hash();
|
|
}
|
|
|
|
if (value.is_bigint())
|
|
return value.as_bigint().big_integer().hash();
|
|
|
|
if (value.is_negative_zero())
|
|
value = Value(0);
|
|
// In the IEEE 754 standard a NaN value is encoded as any value from 0x7ff0000000000001 to 0x7fffffffffffffff,
|
|
// with the least significant bits (referred to as the 'payload') carrying some kind of diagnostic information
|
|
// indicating the source of the NaN. Since ECMA262 does not differentiate between different kinds of NaN values,
|
|
// Sets and Maps must not differentiate between them either.
|
|
// This is achieved by replacing any NaN value by a canonical qNaN.
|
|
else if (value.is_nan())
|
|
value = js_nan();
|
|
|
|
return u64_hash(value.encoded()); // FIXME: Is this the best way to hash pointers, doubles & ints?
|
|
}
|
|
static bool equals(const Value a, const Value b)
|
|
{
|
|
return same_value_zero(a, b);
|
|
}
|
|
};
|
|
|
|
}
|