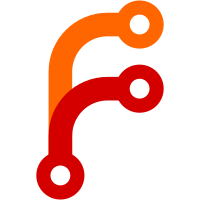
Add classes ExplicitTrackSizing and MetaGridTrackSize which will allow for managing properties like auto-fill and minmax. In the following CSS example there are 3 classes that will be used: grid-template-column: repeat(auto-fill, minmax(50px, 1fr) 75px); ExplicitTrackSizing - will contain the entire value. e.g. repeat(auto-fill, minmax(50px, 1fr) 75px) With a flag if it's a repeat, as well as references to the MetaGridTrackSizes which is the next step down. MetaGridTrackSize: Contain the individual grid track sizes. Here there are two: minmax(50px, 1fr) as well as 75px. This way can keep track if it's a minmax function or not, and the references to both GridTrackSizes in the case it is, or in just the one if it is not. GridTrackSize: Is the most basic element, in this case there are three in total; two of which are held by the first MetaGridTrackSize, and the third is held by the second MetaGridTrackSize. Examples: 50px, 1fr and 75px.
107 lines
2.5 KiB
C++
107 lines
2.5 KiB
C++
/*
|
|
* Copyright (c) 2022, Martin Falisse <mfalisse@outlook.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "GridTrackSize.h"
|
|
#include <AK/String.h>
|
|
#include <LibWeb/CSS/Length.h>
|
|
#include <LibWeb/CSS/Percentage.h>
|
|
#include <LibWeb/CSS/StyleValue.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
GridTrackSize::GridTrackSize(Length length)
|
|
: m_type(Type::Length)
|
|
, m_length(length)
|
|
{
|
|
}
|
|
|
|
GridTrackSize::GridTrackSize(Percentage percentage)
|
|
: m_type(Type::Percentage)
|
|
, m_length { Length::make_px(0) }
|
|
, m_percentage(percentage)
|
|
{
|
|
}
|
|
|
|
GridTrackSize::GridTrackSize(float flexible_length)
|
|
: m_type(Type::FlexibleLength)
|
|
, m_length { Length::make_px(0) }
|
|
, m_flexible_length(flexible_length)
|
|
{
|
|
}
|
|
|
|
GridTrackSize::~GridTrackSize() = default;
|
|
|
|
GridTrackSize GridTrackSize::make_auto()
|
|
{
|
|
return GridTrackSize(CSS::Length::make_auto());
|
|
}
|
|
|
|
String GridTrackSize::to_string() const
|
|
{
|
|
switch (m_type) {
|
|
case Type::Length:
|
|
return m_length.to_string();
|
|
case Type::Percentage:
|
|
return m_percentage.to_string();
|
|
case Type::FlexibleLength:
|
|
return String::formatted("{}fr", m_flexible_length);
|
|
}
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
|
|
Length GridTrackSize::length() const
|
|
{
|
|
return m_length;
|
|
}
|
|
|
|
MetaGridTrackSize::MetaGridTrackSize(GridTrackSize grid_track_size)
|
|
: m_min_grid_track_size(grid_track_size)
|
|
, m_max_grid_track_size(grid_track_size)
|
|
{
|
|
}
|
|
|
|
String MetaGridTrackSize::to_string() const
|
|
{
|
|
return String::formatted("{}", m_min_grid_track_size.to_string());
|
|
}
|
|
|
|
ExplicitTrackSizing::ExplicitTrackSizing()
|
|
{
|
|
}
|
|
|
|
ExplicitTrackSizing::ExplicitTrackSizing(Vector<CSS::MetaGridTrackSize> meta_grid_track_sizes)
|
|
: m_meta_grid_track_sizes(meta_grid_track_sizes)
|
|
{
|
|
}
|
|
|
|
ExplicitTrackSizing::ExplicitTrackSizing(Vector<CSS::MetaGridTrackSize> meta_grid_track_sizes, int repeat_count)
|
|
: m_meta_grid_track_sizes(meta_grid_track_sizes)
|
|
, m_is_repeat(true)
|
|
, m_repeat_count(repeat_count)
|
|
{
|
|
}
|
|
|
|
String ExplicitTrackSizing::to_string() const
|
|
{
|
|
StringBuilder builder;
|
|
if (m_is_repeat) {
|
|
builder.append("repeat("sv);
|
|
builder.append(m_repeat_count);
|
|
builder.append(", "sv);
|
|
}
|
|
for (int _ = 0; _ < m_repeat_count; ++_) {
|
|
for (size_t y = 0; y < m_meta_grid_track_sizes.size(); ++y) {
|
|
builder.append(m_meta_grid_track_sizes[y].to_string());
|
|
if (y != m_meta_grid_track_sizes.size() - 1)
|
|
builder.append(" "sv);
|
|
}
|
|
}
|
|
if (m_is_repeat)
|
|
builder.append(")"sv);
|
|
return builder.to_string();
|
|
}
|
|
|
|
}
|