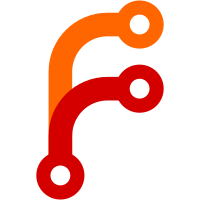
After this commit, Parser::index_of_node_at will prefer to return nodes with greater indices. Since the parsing logic ensures that child nodes come after parent nodes, this change makes this function return child nodes when possible.
27 lines
590 B
C++
27 lines
590 B
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "Token.h"
|
|
|
|
namespace Cpp {
|
|
|
|
bool Position::operator<(const Position& other) const
|
|
{
|
|
return line < other.line || (line == other.line && column < other.column);
|
|
}
|
|
bool Position::operator>(const Position& other) const
|
|
{
|
|
return !(*this < other) && !(*this == other);
|
|
}
|
|
bool Position::operator==(const Position& other) const
|
|
{
|
|
return line == other.line && column == other.column;
|
|
}
|
|
bool Position::operator<=(const Position& other) const
|
|
{
|
|
return !(*this > other);
|
|
}
|
|
}
|