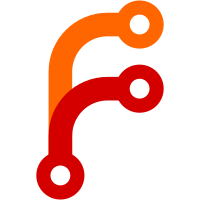
Ownership of the drag data store is a bit weird. In a normal drag-and- drop operation, the DragAndDropEventHandler owns the store. When events are fired for the operation, the DataTransfer object assigned to those events are "associated" with the store. We currently represent that with an Optional<DragDataStore&>. However, it's also possible to create DataTransfer objects from scripts. Those objects create their own drag data store. This puts DataTransfer in a weird situation where it may own a store or just reference one. Rather than coming up with something like Variant<DDS, DDS&> or using MaybeOwned<DDS> here, we can get by with just making the store reference counted.
34 lines
696 B
C++
34 lines
696 B
C++
/*
|
|
* Copyright (c) 2024, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibWeb/HTML/DataTransfer.h>
|
|
#include <LibWeb/HTML/DragDataStore.h>
|
|
|
|
namespace Web::HTML {
|
|
|
|
NonnullRefPtr<DragDataStore> DragDataStore::create()
|
|
{
|
|
return adopt_ref(*new DragDataStore());
|
|
}
|
|
|
|
DragDataStore::DragDataStore()
|
|
: m_allowed_effects_state(DataTransferEffect::uninitialized)
|
|
{
|
|
}
|
|
|
|
DragDataStore::~DragDataStore() = default;
|
|
|
|
bool DragDataStore::has_text_item() const
|
|
{
|
|
for (auto const& item : m_item_list) {
|
|
if (item.kind == DragDataStoreItem::Kind::Text && item.type_string == "text/plain"sv)
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
}
|