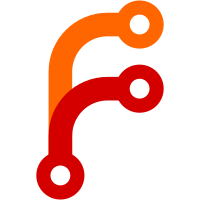
The goal here is to generate most of this code from IPC protocol descriptions, but for now I've spelled them all out to get started. Each message gets a wrapper class in the ASAPI_Client or ASAPI_Server namespace. They are convertible to and from the old message structs. The real hotness happens when you want to make a synchronous request to the other side: auto response = send_sync<ASAPI_Client::GetMainMixVolume>(); Each request class knows his corresponding response class, so in the above example, "response" will be an ASAPI_Server::DidGetMainMixVolume object, and we can get the volume like so: int volume = response.volume(); For posting messages that don't expect a response, you can still use post_message() since the message classes are convertible: post_message(ASAPI_Server::DidGetMainMixVolume(volume)); It's not perfect yet, but I already really like it. :^)
36 lines
923 B
C++
36 lines
923 B
C++
#include <LibAudio/ABuffer.h>
|
|
#include <LibAudio/AClientConnection.h>
|
|
#include <SharedBuffer.h>
|
|
|
|
AClientConnection::AClientConnection()
|
|
: Connection("/tmp/asportal")
|
|
{
|
|
}
|
|
|
|
void AClientConnection::handshake()
|
|
{
|
|
auto response = send_sync<ASAPI_Client::Greeting>(getpid());
|
|
set_server_pid(response.server_pid());
|
|
set_my_client_id(response.your_client_id());
|
|
}
|
|
|
|
void AClientConnection::enqueue(const ABuffer& buffer)
|
|
{
|
|
for (;;) {
|
|
const_cast<ABuffer&>(buffer).shared_buffer().share_with(server_pid());
|
|
auto response = send_sync<ASAPI_Client::EnqueueBuffer>(buffer.shared_buffer_id());
|
|
if (response.success())
|
|
break;
|
|
sleep(1);
|
|
}
|
|
}
|
|
|
|
int AClientConnection::get_main_mix_volume()
|
|
{
|
|
return send_sync<ASAPI_Client::GetMainMixVolume>().volume();
|
|
}
|
|
|
|
void AClientConnection::set_main_mix_volume(int volume)
|
|
{
|
|
send_sync<ASAPI_Client::SetMainMixVolume>(volume);
|
|
}
|