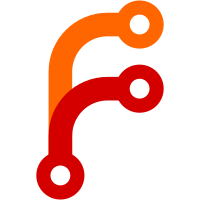
The mutation algorithms now more closely follow the spec and fixes some assertion failures in tests such as Acid3 and Dromaeo. The main thing that is missing right now is passing exceptions to the bindings layer. This is because of issue #6075. I spent a while trying to work it out and got so frustrated I just left it as a FIXME. Besides that, the algorithms bail at the appropriate points. This also makes the adopting steps in the document more spec compliant as it's needed by the insertion algorithm. While I was at it, I added the adoptNode IDL binding. This adds a bunch of ancestor/descendant checks to TreeNode as well. I moved the "remove_all_children" function to Node as it needs to use the full remove algorithm instead of simply removing it from the child list.
34 lines
1.2 KiB
Text
34 lines
1.2 KiB
Text
interface Node : EventTarget {
|
|
|
|
readonly attribute unsigned short nodeType;
|
|
readonly attribute DOMString nodeName;
|
|
|
|
boolean hasChildNodes();
|
|
// FIXME: This should be a NodeList
|
|
readonly attribute ArrayFromVector childNodes;
|
|
readonly attribute Node? firstChild;
|
|
readonly attribute Node? lastChild;
|
|
readonly attribute Node? previousSibling;
|
|
readonly attribute Node? nextSibling;
|
|
readonly attribute Node? parentNode;
|
|
readonly attribute Element? parentElement;
|
|
attribute DOMString textContent;
|
|
|
|
Node appendChild(Node node);
|
|
[ImplementedAs=pre_insert] Node insertBefore(Node node, Node? child);
|
|
[ImplementedAs=pre_remove] Node removeChild(Node child);
|
|
|
|
const unsigned short ELEMENT_NODE = 1;
|
|
const unsigned short ATTRIBUTE_NODE = 2;
|
|
const unsigned short TEXT_NODE = 3;
|
|
const unsigned short CDATA_SECTION_NODE = 4;
|
|
const unsigned short ENTITY_REFERENCE_NODE = 5;
|
|
const unsigned short ENTITY_NODE = 6;
|
|
const unsigned short PROCESSING_INSTRUCTION_NODE = 7;
|
|
const unsigned short COMMENT_NODE = 8;
|
|
const unsigned short DOCUMENT_NODE = 9;
|
|
const unsigned short DOCUMENT_TYPE_NODE = 10;
|
|
const unsigned short DOCUMENT_FRAGMENT_NODE = 11;
|
|
const unsigned short NOTATION_NODE = 12;
|
|
|
|
};
|