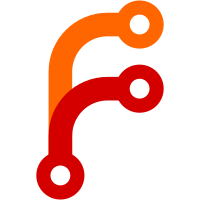
This renames Object::to_primitive() to Object::ordinary_to_primitive() for two reasons: - No confusion with Value::to_primitive() - To match the spec's name Also change existing uses of Object::to_primitive() to Value::to_primitive() when the spec uses the latter (which will still call Object::ordinary_to_primitive()). Object::to_string() has been removed as it's not needed anymore (and nothing the spec uses). This makes it possible to overwrite an object's toString and valueOf and have them provide results for anything that uses to_primitive() - e.g.: const o = { toString: undefined, valueOf: () => 42 }; Number(o) // 42, previously NaN ["foo", o].toString(); // "foo,42", previously "foo,[object Object]" ++o // 43, previously NaN etc.
23 lines
740 B
JavaScript
23 lines
740 B
JavaScript
test("object with custom toString", () => {
|
|
const o = { toString: () => "foo" };
|
|
expect(o + "bar").toBe("foobar");
|
|
expect([o, "bar"].toString()).toBe("foo,bar");
|
|
});
|
|
|
|
test("object with uncallable toString and custom valueOf", () => {
|
|
const o = { toString: undefined, valueOf: () => "foo" };
|
|
expect(o + "bar").toBe("foobar");
|
|
expect([o, "bar"].toString()).toBe("foo,bar");
|
|
});
|
|
|
|
test("object with custom valueOf", () => {
|
|
const o = { valueOf: () => 42 };
|
|
expect(Number(o)).toBe(42);
|
|
expect(o + 1).toBe(43);
|
|
});
|
|
|
|
test("object with uncallable valueOf and custom toString", () => {
|
|
const o = { valueOf: undefined, toString: () => "42" };
|
|
expect(Number(o)).toBe(42);
|
|
expect(o + 1).toBe("421");
|
|
});
|