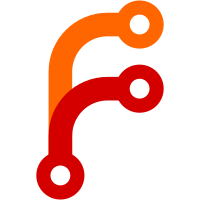
Per the spec, only a BlockContainer" can have line boxes, so let's not clutter up every Layout::Box with line boxes. This also allows us to establish an invariant that BFC and IFC always operate on a Layout::BlockContainer. Note that if BlockContainer has all block-level children, its line boxes are not used for anything. They are only used in the all inline-level children scenario.
31 lines
861 B
C++
31 lines
861 B
C++
/*
|
|
* Copyright (c) 2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Types.h>
|
|
#include <LibWeb/Forward.h>
|
|
#include <LibWeb/Layout/BlockContainer.h>
|
|
#include <LibWeb/Layout/FormattingContext.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
class InlineFormattingContext final : public FormattingContext {
|
|
public:
|
|
InlineFormattingContext(BlockContainer& containing_block, FormattingContext* parent);
|
|
~InlineFormattingContext();
|
|
|
|
BlockContainer& containing_block() { return static_cast<BlockContainer&>(context_box()); }
|
|
BlockContainer const& containing_block() const { return static_cast<BlockContainer const&>(context_box()); }
|
|
|
|
virtual void run(Box&, LayoutMode) override;
|
|
|
|
float available_width_at_line(size_t line_index) const;
|
|
|
|
void dimension_box_on_line(Box&, LayoutMode);
|
|
};
|
|
|
|
}
|