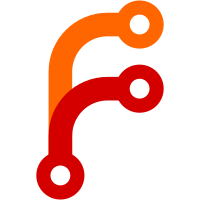
This change makes WebGL to use LibGL only in SerenityOS, and the platform's OpenGL driver in Ladybird if it is available. This is implemented by introducing wrapper class between WebGL and OpenGL calls. This way it will also be possible to provide more complete support in Ladybird even if we don't yet have all needed calls implemented in LibGL. For now, the wrapper class makes all GL calls virtual. However, we can get rid of this and implement it at compile time in case of performance problems.
32 lines
1 KiB
C++
32 lines
1 KiB
C++
/*
|
|
* Copyright (c) 2022, Luke Wilde <lukew@serenityos.org>
|
|
* Copyright (c) 2024, Aliaksandr Kalenik <kalenik.aliaksandr@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/OwnPtr.h>
|
|
#include <LibJS/Heap/GCPtr.h>
|
|
#include <LibWeb/Bindings/PlatformObject.h>
|
|
#include <LibWeb/WebGL/WebGLRenderingContextBase.h>
|
|
|
|
namespace Web::WebGL {
|
|
|
|
class WebGLRenderingContext final : public WebGLRenderingContextBase {
|
|
WEB_PLATFORM_OBJECT(WebGLRenderingContext, WebGLRenderingContextBase);
|
|
JS_DECLARE_ALLOCATOR(WebGLRenderingContext);
|
|
|
|
public:
|
|
static JS::ThrowCompletionOr<JS::GCPtr<WebGLRenderingContext>> create(JS::Realm&, HTML::HTMLCanvasElement& canvas_element, JS::Value options);
|
|
|
|
virtual ~WebGLRenderingContext() override;
|
|
|
|
private:
|
|
virtual void initialize(JS::Realm&) override;
|
|
|
|
WebGLRenderingContext(JS::Realm&, HTML::HTMLCanvasElement&, NonnullOwnPtr<OpenGLContext> context, WebGLContextAttributes context_creation_parameters, WebGLContextAttributes actual_context_parameters);
|
|
};
|
|
|
|
}
|