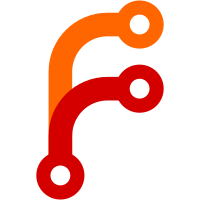
With this change, we now have ~1200 CellAllocators across both LibJS and LibWeb in a normal WebContent instance. This gives us a minimum heap size of 4.7 MiB in the scenario where we only have one cell allocated per type. Of course, in practice there will be many more of each type, so the effective overhead is quite a bit smaller than that in practice. I left a few types unconverted to this mechanism because I got tired of doing this. :^)
67 lines
2.2 KiB
C++
67 lines
2.2 KiB
C++
/*
|
|
* Copyright (c) 2023, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibWeb/Bindings/Intrinsics.h>
|
|
#include <LibWeb/HTML/HTMLDetailsElement.h>
|
|
#include <LibWeb/HTML/HTMLSummaryElement.h>
|
|
|
|
namespace Web::HTML {
|
|
|
|
JS_DEFINE_ALLOCATOR(HTMLSummaryElement);
|
|
|
|
HTMLSummaryElement::HTMLSummaryElement(DOM::Document& document, DOM::QualifiedName qualified_name)
|
|
: HTMLElement(document, move(qualified_name))
|
|
{
|
|
activation_behavior = [this](auto&) {
|
|
// The activation behavior of summary elements is to run the following steps:
|
|
|
|
// 1. If this summary element is not the summary for its parent details, then return.
|
|
if (!is_summary_for_its_parent_details())
|
|
return;
|
|
|
|
// 2. Let parent be this summary element's parent.
|
|
auto* parent = this->parent_element();
|
|
|
|
// 3. If the open attribute is present on parent, then remove it. Otherwise, set parent's open attribute to the empty string.
|
|
if (parent->has_attribute(HTML::AttributeNames::open))
|
|
parent->remove_attribute(HTML::AttributeNames::open);
|
|
else
|
|
parent->set_attribute(HTML::AttributeNames::open, String {}).release_value_but_fixme_should_propagate_errors();
|
|
};
|
|
}
|
|
|
|
// https://html.spec.whatwg.org/multipage/interactive-elements.html#summary-for-its-parent-details
|
|
bool HTMLSummaryElement::is_summary_for_its_parent_details()
|
|
{
|
|
// A summary element is a summary for its parent details if the following algorithm returns true:
|
|
|
|
// 1. If this summary element has no parent, then return false.
|
|
if (!parent_element())
|
|
return false;
|
|
|
|
// 2. Let parent be this summary element's parent.
|
|
auto* parent = this->parent_element();
|
|
|
|
// 3. If parent is not a details element, then return false.
|
|
if (!is<HTMLDetailsElement>(*parent))
|
|
return false;
|
|
|
|
// 4. If parent's first summary element child is not this summary element, then return false.
|
|
if (parent->first_child_of_type<HTMLSummaryElement>() != this)
|
|
return false;
|
|
|
|
// 5. Return true.
|
|
return true;
|
|
}
|
|
|
|
HTMLSummaryElement::~HTMLSummaryElement() = default;
|
|
|
|
void HTMLSummaryElement::initialize(JS::Realm& realm)
|
|
{
|
|
Base::initialize(realm);
|
|
}
|
|
|
|
}
|