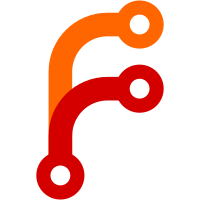
This basically adds the line u8 token = TRY( tree_decode(decoder, COEFFICIENT_TREE, header.coefficient_probabilities[plane][band][tricky], last_decoded_value == DCT_0 ? 2 : 0)); and calls it once for the 16 coefficients of a discrete cosine transform that covers a 4x4 pixel subblock. And then it does this 24 or 25 times per macroblock, for the 4x4 Y subblocks and the 2x2 each U and V subblocks (plus an optional Y2 block for some macroblocks). It then adds a whole bunch of machinery to be able to compute `plane`, `band`, and in particular `tricky` (which depends on if the corresponding left or above subblock has non-zero coefficients). (It also does dequantization, and does an inverse Walsh-Hadamard transform when needed, to end up with complete DCT coefficients in all cases.)
32 lines
762 B
C++
32 lines
762 B
C++
/*
|
|
* Copyright (c) 2023, Nico Weber <thakis@chromium.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Span.h>
|
|
#include <AK/Types.h>
|
|
#include <LibGfx/Bitmap.h>
|
|
|
|
namespace Gfx {
|
|
|
|
struct VP8Header {
|
|
u8 version;
|
|
bool show_frame;
|
|
u32 size_of_first_partition;
|
|
u32 width;
|
|
u8 horizontal_scale;
|
|
u32 height;
|
|
u8 vertical_scale;
|
|
ReadonlyBytes first_partition;
|
|
ReadonlyBytes second_partition;
|
|
};
|
|
|
|
// Parses the header data in a VP8 chunk. Pass the payload of a `VP8 ` chunk, after the tag and after the tag's data size.
|
|
ErrorOr<VP8Header> decode_webp_chunk_VP8_header(ReadonlyBytes vp8_data);
|
|
|
|
ErrorOr<NonnullRefPtr<Bitmap>> decode_webp_chunk_VP8_contents(VP8Header const&, bool include_alpha_channel);
|
|
|
|
}
|