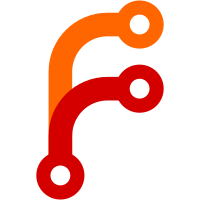
The old name is the result of the perhaps somewhat confusingly named abstract operation OrdinaryFunctionCreate(), which creates an "ordinary object" (https://tc39.es/ecma262/#ordinary-object) in contrast to an "exotic object" (https://tc39.es/ecma262/#exotic-object). However, the term "Ordinary Function" is not used anywhere in the spec, instead the created object is referred to as an "ECMAScript Function Object" (https://tc39.es/ecma262/#sec-ecmascript-function-objects), so let's call it that. The "ordinary" vs. "exotic" distinction is important because there are also "Built-in Function Objects", which can be either implemented as ordinary ECMAScript function objects, or as exotic objects (our NativeFunction). More work needs to be done to move a lot of infrastructure to ECMAScriptFunctionObject in order to make FunctionObject nothing more than an interface for objects that implement [[Call]] and optionally [[Construct]].
24 lines
743 B
JavaScript
24 lines
743 B
JavaScript
test("basic functionality", () => {
|
|
const localSym = Symbol("foo");
|
|
const globalSym = Symbol.for("foo");
|
|
|
|
expect(Symbol.keyFor(localSym)).toBeUndefined();
|
|
expect(Symbol.keyFor(globalSym)).toBe("foo");
|
|
});
|
|
|
|
test("bad argument values", () => {
|
|
[
|
|
[1, "1"],
|
|
[null, "null"],
|
|
[undefined, "undefined"],
|
|
[[], "[object Array]"],
|
|
[{}, "[object Object]"],
|
|
[true, "true"],
|
|
["foobar", "foobar"],
|
|
[function () {}, "[object ECMAScriptFunctionObject]"], // FIXME: Better function stringification
|
|
].forEach(testCase => {
|
|
expect(() => {
|
|
Symbol.keyFor(testCase[0]);
|
|
}).toThrowWithMessage(TypeError, `${testCase[1]} is not a symbol`);
|
|
});
|
|
});
|