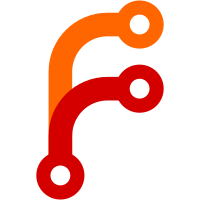
Range's member variables are stored in AbstractRange as per the spec, as they are also shared with StaticRange.
90 lines
2.3 KiB
C++
90 lines
2.3 KiB
C++
/*
|
||
* Copyright (c) 2020, the SerenityOS developers.
|
||
* Copyright (c) 2022, Luke Wilde <lukew@serenityos.org>
|
||
*
|
||
* SPDX-License-Identifier: BSD-2-Clause
|
||
*/
|
||
|
||
#include <LibWeb/DOM/Document.h>
|
||
#include <LibWeb/DOM/Node.h>
|
||
#include <LibWeb/DOM/Range.h>
|
||
#include <LibWeb/DOM/Window.h>
|
||
|
||
namespace Web::DOM {
|
||
|
||
NonnullRefPtr<Range> Range::create(Window& window)
|
||
{
|
||
return Range::create(window.associated_document());
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::create(Document& document)
|
||
{
|
||
return adopt_ref(*new Range(document));
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::create(Node& start_container, u32 start_offset, Node& end_container, u32 end_offset)
|
||
{
|
||
return adopt_ref(*new Range(start_container, start_offset, end_container, end_offset));
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::create_with_global_object(Bindings::WindowObject& window)
|
||
{
|
||
return Range::create(window.impl());
|
||
}
|
||
|
||
Range::Range(Document& document)
|
||
: Range(document, 0, document, 0)
|
||
{
|
||
}
|
||
|
||
Range::Range(Node& start_container, u32 start_offset, Node& end_container, u32 end_offset)
|
||
: AbstractRange(start_container, start_offset, end_container, end_offset)
|
||
{
|
||
}
|
||
|
||
Range::~Range()
|
||
{
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::clone_range() const
|
||
{
|
||
return adopt_ref(*new Range(const_cast<Node&>(*m_start_container), m_start_offset, const_cast<Node&>(*m_end_container), m_end_offset));
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::inverted() const
|
||
{
|
||
return adopt_ref(*new Range(const_cast<Node&>(*m_end_container), m_end_offset, const_cast<Node&>(*m_start_container), m_start_offset));
|
||
}
|
||
|
||
NonnullRefPtr<Range> Range::normalized() const
|
||
{
|
||
if (m_start_container.ptr() == m_end_container.ptr()) {
|
||
if (m_start_offset <= m_end_offset)
|
||
return clone_range();
|
||
|
||
return inverted();
|
||
}
|
||
|
||
if (m_start_container->is_before(m_end_container))
|
||
return clone_range();
|
||
|
||
return inverted();
|
||
}
|
||
|
||
// https://dom.spec.whatwg.org/#dom-range-commonancestorcontainer
|
||
NonnullRefPtr<Node> Range::common_ancestor_container() const
|
||
{
|
||
// 1. Let container be start node.
|
||
auto container = m_start_container;
|
||
|
||
// 2. While container is not an inclusive ancestor of end node, let container be container’s parent.
|
||
while (!container->is_inclusive_ancestor_of(m_end_container)) {
|
||
VERIFY(container->parent());
|
||
container = *container->parent();
|
||
}
|
||
|
||
// 3. Return container.
|
||
return container;
|
||
}
|
||
|
||
}
|