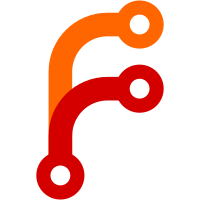
This commit introduces the concept of an accumulator register to LibJS's bytecode interpreter. The accumulator register is always register 0, and most simple instructions use it for reading and writing. Not only does this slim down the AST, but it also simplifies a lot of the code. For example, the generate_bytecode methods no longer need to return an Optional<Register>, as any opcode which has a "return" value will always put it into the accumulator. This also renames the old Op::Load to Op::LoadImmediate, and uses Op::Load to load from a register into the accumulator. There is also an Op::Store to put the value in the accumulator into another register.
44 lines
935 B
C++
44 lines
935 B
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Format.h>
|
|
|
|
namespace JS::Bytecode {
|
|
|
|
class Register {
|
|
public:
|
|
constexpr static u32 accumulator_index = 0;
|
|
|
|
static Register accumulator()
|
|
{
|
|
static Register accumulator(accumulator_index);
|
|
return accumulator;
|
|
}
|
|
|
|
explicit Register(u32 index)
|
|
: m_index(index)
|
|
{
|
|
}
|
|
|
|
u32 index() const { return m_index; }
|
|
|
|
private:
|
|
u32 m_index;
|
|
};
|
|
|
|
}
|
|
|
|
template<>
|
|
struct AK::Formatter<JS::Bytecode::Register> : AK::Formatter<FormatString> {
|
|
void format(FormatBuilder& builder, JS::Bytecode::Register const& value)
|
|
{
|
|
if (value.index() == JS::Bytecode::Register::accumulator_index)
|
|
return AK::Formatter<FormatString>::format(builder, "acc");
|
|
return AK::Formatter<FormatString>::format(builder, "${}", value.index());
|
|
}
|
|
};
|