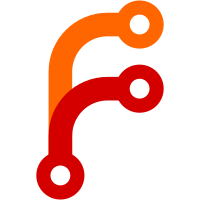
As mentioned on Discord earlier, we'll add these to all new functions going forward - this is the backfill. Reasons: - It makes you look at the spec, implementing based on MDN or V8 behavior is a no-go - It makes finding the various functions that are non-compliant easier, in the future everything should either have such a comment or, if it's not from the spec at all, a comment explaining why that is the case - It makes it easier to check whether a certain abstract operation is implemented in LibJS, not all of them use the same name as the spec. E.g. RejectPromise() is Promise::reject() - It makes it easier to reason about vm.arguments(), e.g. when the function has a rest parameter - It makes it easier to see whether a certain function is from a proposal or Annex B Also: - Add arguments to all functions and abstract operations that already had a comment - Fix some outdated section numbers - Replace some ecma-international.org URLs with tc39.es
34 lines
905 B
C++
34 lines
905 B
C++
/*
|
|
* Copyright (c) 2021, Linus Groh <linusg@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibJS/Runtime/Function.h>
|
|
#include <LibJS/Runtime/VM.h>
|
|
|
|
namespace JS {
|
|
|
|
// 9.5.1 JobCallback Records, https://tc39.es/ecma262/#sec-jobcallback-records
|
|
struct JobCallback {
|
|
Function* callback;
|
|
};
|
|
|
|
// 9.5.2 HostMakeJobCallback ( callback ), https://tc39.es/ecma262/#sec-hostmakejobcallback
|
|
inline JobCallback make_job_callback(Function& callback)
|
|
{
|
|
return { &callback };
|
|
}
|
|
|
|
// 9.5.3 HostCallJobCallback ( jobCallback, V, argumentsList ), https://tc39.es/ecma262/#sec-hostcalljobcallback
|
|
template<typename... Args>
|
|
[[nodiscard]] inline Value call_job_callback(VM& vm, JobCallback& job_callback, Value this_value, Args... args)
|
|
{
|
|
VERIFY(job_callback.callback);
|
|
auto& callback = *job_callback.callback;
|
|
return vm.call(callback, this_value, args...);
|
|
}
|
|
|
|
}
|