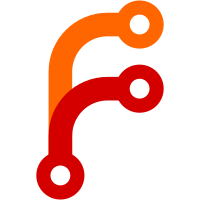
If we determine that a selector is simple enough, we now run it using a special matching loop that traverses up the DOM ancestor chain without recursion. The criteria for this fast path are: - All combinators involved must be either descendant or child. - Only tag name, class, ID and attribute selectors allowed. It's definitely possible to increase the coverage of this fast path, but this first version already provides a substantial reduction in time spent evaluating selectors. 48% of the selectors evaluated when loading our GitHub repo are now using this fast path. 18% speed-up on the "Descendant and child combinators" subtest of StyleBench. :^)
19 lines
642 B
C++
19 lines
642 B
C++
/*
|
|
* Copyright (c) 2018-2024, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/CSS/Selector.h>
|
|
#include <LibWeb/DOM/Element.h>
|
|
|
|
namespace Web::SelectorEngine {
|
|
|
|
bool matches(CSS::Selector const&, Optional<CSS::CSSStyleSheet const&> style_sheet_for_rule, DOM::Element const&, Optional<CSS::Selector::PseudoElement::Type> = {}, JS::GCPtr<DOM::ParentNode const> scope = {});
|
|
|
|
[[nodiscard]] bool fast_matches(CSS::Selector const&, Optional<CSS::CSSStyleSheet const&> style_sheet_for_rule, DOM::Element const&);
|
|
[[nodiscard]] bool can_use_fast_matches(CSS::Selector const&);
|
|
|
|
}
|