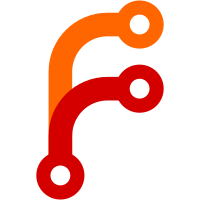
The current implementation felt a bit ad-hoc and notably allowed textContent to operate on all node types. It also only returned the child text content of the Node instead of the descendant text content.
35 lines
698 B
C++
35 lines
698 B
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <LibWeb/DOM/Node.h>
|
|
#include <LibWeb/DOM/NonDocumentTypeChildNode.h>
|
|
|
|
namespace Web::DOM {
|
|
|
|
class CharacterData
|
|
: public Node
|
|
, public NonDocumentTypeChildNode<CharacterData> {
|
|
public:
|
|
using WrapperType = Bindings::CharacterDataWrapper;
|
|
|
|
virtual ~CharacterData() override;
|
|
|
|
const String& data() const { return m_data; }
|
|
void set_data(String);
|
|
|
|
unsigned length() const { return m_data.length(); }
|
|
|
|
protected:
|
|
explicit CharacterData(Document&, NodeType, const String&);
|
|
|
|
private:
|
|
String m_data;
|
|
};
|
|
|
|
}
|