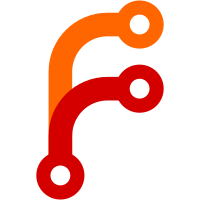
Previously these were all passed around by value, but some of them (StyleComponentValueRule and StyleBlockRule) want to include each other as fields, so this had to change.
61 lines
1.3 KiB
C++
61 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, the SerenityOS developers.
|
|
* Copyright (c) 2021, Sam Atkins <atkinssj@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <AK/RefPtr.h>
|
|
#include <LibWeb/CSS/Parser/Token.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
class StyleBlockRule;
|
|
class StyleFunctionRule;
|
|
|
|
class StyleComponentValueRule {
|
|
friend class Parser;
|
|
|
|
public:
|
|
enum class ComponentType {
|
|
Token,
|
|
Function,
|
|
Block
|
|
};
|
|
|
|
explicit StyleComponentValueRule(ComponentType);
|
|
~StyleComponentValueRule();
|
|
|
|
bool is_block() const { return m_type == ComponentType::Block; }
|
|
StyleBlockRule const& block() const
|
|
{
|
|
VERIFY(is_block());
|
|
return *m_block;
|
|
}
|
|
|
|
bool is_function() const { return m_type == ComponentType::Function; }
|
|
StyleFunctionRule const& function() const
|
|
{
|
|
VERIFY(is_function());
|
|
return *m_function;
|
|
}
|
|
|
|
bool is(Token::TokenType type) const
|
|
{
|
|
return m_type == ComponentType::Token && m_token.is(type);
|
|
}
|
|
Token const& token() const { return m_token; }
|
|
operator Token() const { return m_token; }
|
|
|
|
String to_string() const;
|
|
|
|
private:
|
|
ComponentType m_type;
|
|
Token m_token;
|
|
RefPtr<StyleFunctionRule> m_function;
|
|
RefPtr<StyleBlockRule> m_block;
|
|
};
|
|
}
|