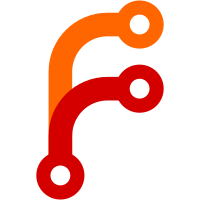
getComputedStyle(element) now returns a ComputedCSSStyleDeclaration object, which is a live view of the computed style of a given element. This works by ComputedCSSStyleDeclaration being a wrapper around an element pointer. When you ask it for a CSS property, it gets the latest computed style values from the element and returns them as a CSS::StyleProperty object. This first cut adds support for computed 'color' and 'display'. In case the element doesn't have a corresponding node in the layout tree, we fall back to using specified style instead. This is achieved by performing an on-the-fly style resolution for the individual element and then grabbing the requested property from that resolved style.
33 lines
853 B
C++
33 lines
853 B
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/CSS/CSSStyleDeclaration.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
class ComputedCSSStyleDeclaration final : public CSSStyleDeclaration {
|
|
public:
|
|
static NonnullRefPtr<ComputedCSSStyleDeclaration> create(DOM::Element& element)
|
|
{
|
|
return adopt_ref(*new ComputedCSSStyleDeclaration(element));
|
|
}
|
|
|
|
virtual ~ComputedCSSStyleDeclaration() override;
|
|
|
|
virtual size_t length() const override;
|
|
virtual String item(size_t index) const override;
|
|
virtual Optional<StyleProperty> property(PropertyID) const override;
|
|
virtual bool set_property(PropertyID, StringView css_text) override;
|
|
|
|
private:
|
|
explicit ComputedCSSStyleDeclaration(DOM::Element&);
|
|
|
|
NonnullRefPtr<DOM::Element> m_element;
|
|
};
|
|
|
|
}
|