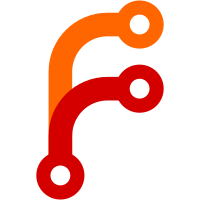
This patch begins the support for the 'view-box' attribute that can be attached to <svg>'s. The FormattingContext determines the size of the Element according to the specified 'width' and 'height' or if they are not given by the 'viewbox' or by the bounding box of the path if nothing is specified. When we try to paint a SVG Path that belongs to a <svg> that has the 'view-box' and a specified 'height'/'width', all the parts of the path get scaled/moved accordingly. There probably are many edge cases and bugs still to be found, but this is a nice start. :^)
34 lines
1 KiB
C++
34 lines
1 KiB
C++
/*
|
|
* Copyright (c) 2020, Matthew Olsson <mattco@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Layout/SVGGraphicsBox.h>
|
|
#include <LibWeb/SVG/SVGGeometryElement.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
class SVGGeometryBox final : public SVGGraphicsBox {
|
|
public:
|
|
SVGGeometryBox(DOM::Document&, SVG::SVGGeometryElement&, NonnullRefPtr<CSS::StyleProperties>);
|
|
virtual ~SVGGeometryBox() override = default;
|
|
|
|
SVG::SVGGeometryElement& dom_node() { return verify_cast<SVG::SVGGeometryElement>(SVGGraphicsBox::dom_node()); }
|
|
SVG::SVGGeometryElement const& dom_node() const { return verify_cast<SVG::SVGGeometryElement>(SVGGraphicsBox::dom_node()); }
|
|
|
|
virtual void paint(PaintContext& context, PaintPhase phase) override;
|
|
|
|
float viewbox_scaling() const;
|
|
Gfx::FloatPoint viewbox_origin() const;
|
|
|
|
private:
|
|
virtual bool is_svg_geometry_box() const final { return true; }
|
|
};
|
|
|
|
template<>
|
|
inline bool Node::fast_is<SVGGeometryBox>() const { return is_svg_geometry_box(); }
|
|
|
|
}
|