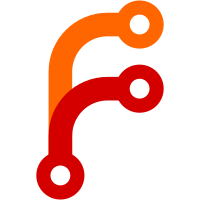
Instead of each card being responsible for painting its own bitmaps, we now have a CardPainter which is responsible for this. It paints a given card the first time it is requested, and then re-uses that bitmap when requested in the future. This saves memory for duplicate cards (such as in Spider where several sets of the same suit are used) or unused ones (for example, the inverted cards which are only used by Hearts). It also means we don't throw away bitmaps and then re-create identical ones when starting a new game. We get some nice memory savings from this: | | Before | After | Before (Virtual) | After (Virtual) | |:----------|---------:|---------:|-----------------:|----------------:| | Hearts | 12.2 MiB | 9.3 MiB | 25.1 MiB | 22.2 MiB | | Spider | 12.1 MiB | 10.1 MiB | 29.2 MiB | 22.9 MiB | | Solitaire | 16.4 MiB | 9.0 MiB | 25.0 MiB | 21.9 MiB | All these measurements taken from x86_64 build, from a fresh launch of each game after the animation has finished, but without making any moves. The Hearts value will go up once inverted cards start being requested.
203 lines
5.7 KiB
C++
203 lines
5.7 KiB
C++
/*
|
|
* Copyright (c) 2020, Till Mayer <till.mayer@web.de>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
* Copyright (c) 2022, Sam Atkins <atkinssj@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "CardPainter.h"
|
|
#include <LibGfx/Font/Font.h>
|
|
#include <LibGfx/Font/FontDatabase.h>
|
|
|
|
namespace Cards {
|
|
|
|
CardPainter& CardPainter::the()
|
|
{
|
|
static CardPainter s_card_painter;
|
|
return s_card_painter;
|
|
}
|
|
|
|
static constexpr Gfx::CharacterBitmap s_diamond {
|
|
" # "
|
|
" ### "
|
|
" ##### "
|
|
" ####### "
|
|
"#########"
|
|
" ####### "
|
|
" ##### "
|
|
" ### "
|
|
" # "sv,
|
|
9, 9
|
|
};
|
|
|
|
static constexpr Gfx::CharacterBitmap s_heart {
|
|
" # # "
|
|
" ### ### "
|
|
"#########"
|
|
"#########"
|
|
"#########"
|
|
" ####### "
|
|
" ##### "
|
|
" ### "
|
|
" # "sv,
|
|
9, 9
|
|
};
|
|
|
|
static constexpr Gfx::CharacterBitmap s_spade {
|
|
" # "
|
|
" ### "
|
|
" ##### "
|
|
" ####### "
|
|
"#########"
|
|
"#########"
|
|
" ## # ## "
|
|
" ### "
|
|
" ### "sv,
|
|
9, 9
|
|
};
|
|
|
|
static constexpr Gfx::CharacterBitmap s_club {
|
|
" ### "
|
|
" ##### "
|
|
" ##### "
|
|
" ## ### ## "
|
|
"###########"
|
|
"###########"
|
|
"#### # ####"
|
|
" ## ### ## "
|
|
" ### "sv,
|
|
11, 9
|
|
};
|
|
|
|
NonnullRefPtr<Gfx::Bitmap> CardPainter::card_front(Suit suit, Rank rank)
|
|
{
|
|
auto suit_id = to_underlying(suit);
|
|
auto rank_id = to_underlying(rank);
|
|
|
|
auto& existing_bitmap = m_cards[suit_id][rank_id];
|
|
if (!existing_bitmap.is_null())
|
|
return *existing_bitmap;
|
|
|
|
m_cards[suit_id][rank_id] = create_card_bitmap();
|
|
paint_card_front(*m_cards[suit_id][rank_id], suit, rank);
|
|
|
|
return *m_cards[suit_id][rank_id];
|
|
}
|
|
|
|
NonnullRefPtr<Gfx::Bitmap> CardPainter::card_back()
|
|
{
|
|
if (!m_card_back.is_null())
|
|
return *m_card_back;
|
|
|
|
m_card_back = create_card_bitmap();
|
|
paint_card_back(*m_card_back);
|
|
|
|
return *m_card_back;
|
|
}
|
|
|
|
NonnullRefPtr<Gfx::Bitmap> CardPainter::card_front_inverted(Suit suit, Rank rank)
|
|
{
|
|
auto suit_id = to_underlying(suit);
|
|
auto rank_id = to_underlying(rank);
|
|
|
|
auto& existing_bitmap = m_cards_inverted[suit_id][rank_id];
|
|
if (!existing_bitmap.is_null())
|
|
return *existing_bitmap;
|
|
|
|
m_cards_inverted[suit_id][rank_id] = create_card_bitmap();
|
|
paint_inverted_card(*m_cards_inverted[suit_id][rank_id], card_front(suit, rank));
|
|
|
|
return *m_cards_inverted[suit_id][rank_id];
|
|
}
|
|
|
|
NonnullRefPtr<Gfx::Bitmap> CardPainter::card_back_inverted()
|
|
{
|
|
if (!m_card_back_inverted.is_null())
|
|
return *m_card_back_inverted;
|
|
|
|
m_card_back_inverted = create_card_bitmap();
|
|
paint_inverted_card(card_back(), *m_card_back_inverted);
|
|
|
|
return *m_card_back_inverted;
|
|
}
|
|
|
|
NonnullRefPtr<Gfx::Bitmap> CardPainter::create_card_bitmap()
|
|
{
|
|
return Gfx::Bitmap::try_create(Gfx::BitmapFormat::BGRA8888, { Card::width, Card::height }).release_value_but_fixme_should_propagate_errors();
|
|
}
|
|
|
|
void CardPainter::paint_card_front(Gfx::Bitmap& bitmap, Cards::Suit suit, Cards::Rank rank)
|
|
{
|
|
auto const suit_color = (suit == Suit::Diamonds || suit == Suit::Hearts) ? Color::Red : Color::Black;
|
|
|
|
auto const& suit_symbol = [&]() -> Gfx::CharacterBitmap const& {
|
|
switch (suit) {
|
|
case Suit::Diamonds:
|
|
return s_diamond;
|
|
case Suit::Clubs:
|
|
return s_club;
|
|
case Suit::Spades:
|
|
return s_spade;
|
|
case Suit::Hearts:
|
|
return s_heart;
|
|
default:
|
|
VERIFY_NOT_REACHED();
|
|
}
|
|
}();
|
|
|
|
Gfx::Painter painter { bitmap };
|
|
auto paint_rect = bitmap.rect();
|
|
auto& font = Gfx::FontDatabase::default_font().bold_variant();
|
|
|
|
painter.fill_rect_with_rounded_corners(paint_rect, Color::Black, Card::card_radius);
|
|
paint_rect.shrink(2, 2);
|
|
painter.fill_rect_with_rounded_corners(paint_rect, Color::White, Card::card_radius - 1);
|
|
|
|
paint_rect.set_height(paint_rect.height() / 2);
|
|
paint_rect.shrink(10, 6);
|
|
|
|
auto text_rect = Gfx::IntRect { 4, 6, font.width("10"sv), font.glyph_height() };
|
|
painter.draw_text(text_rect, card_rank_label(rank), font, Gfx::TextAlignment::Center, suit_color);
|
|
|
|
painter.draw_bitmap(
|
|
{ text_rect.x() + (text_rect.width() - suit_symbol.size().width()) / 2, text_rect.bottom() + 5 },
|
|
suit_symbol, suit_color);
|
|
|
|
for (int y = Card::height / 2; y < Card::height; ++y) {
|
|
for (int x = 0; x < Card::width; ++x) {
|
|
bitmap.set_pixel(x, y, bitmap.get_pixel(Card::width - x - 1, Card::height - y - 1));
|
|
}
|
|
}
|
|
}
|
|
|
|
void CardPainter::paint_card_back(Gfx::Bitmap& bitmap)
|
|
{
|
|
Gfx::Painter painter { bitmap };
|
|
auto paint_rect = bitmap.rect();
|
|
painter.clear_rect(paint_rect, Gfx::Color::Transparent);
|
|
|
|
auto image = Gfx::Bitmap::try_load_from_file("/res/icons/cards/buggie-deck.png"sv).release_value_but_fixme_should_propagate_errors();
|
|
|
|
float aspect_ratio = image->width() / static_cast<float>(image->height());
|
|
Gfx::IntSize target_size { static_cast<int>(aspect_ratio * (Card::height - 5)), Card::height - 5 };
|
|
|
|
painter.fill_rect_with_rounded_corners(paint_rect, Color::Black, Card::card_radius);
|
|
auto inner_paint_rect = paint_rect.shrunken(2, 2);
|
|
painter.fill_rect_with_rounded_corners(inner_paint_rect, Color::White, Card::card_radius - 1);
|
|
|
|
painter.draw_scaled_bitmap(
|
|
{ { (Card::width - target_size.width()) / 2, (Card::height - target_size.height()) / 2 }, target_size },
|
|
*image, image->rect());
|
|
}
|
|
|
|
void CardPainter::paint_inverted_card(Gfx::Bitmap& bitmap, Gfx::Bitmap const& source_to_invert)
|
|
{
|
|
Gfx::Painter painter { bitmap };
|
|
painter.clear_rect(bitmap.rect(), Gfx::Color::Transparent);
|
|
painter.blit_filtered({ 0, 0 }, source_to_invert, source_to_invert.rect(), [&](Color color) {
|
|
return color.inverted();
|
|
});
|
|
}
|
|
|
|
}
|