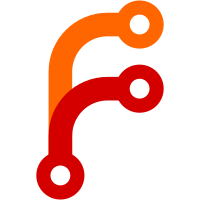
This is a continuation of the previous two commits. As allocating a JS cell already primarily involves a realm instead of a global object, and we'll need to pass one to the allocate() function itself eventually (it's bridged via the global object right now), the create() functions need to receive a realm as well. The plan is for this to be the highest-level function that actually receives a realm and passes it around, AOs on an even higher level will use the "current realm" concept via VM::current_realm() as that's what the spec assumes; passing around realms (or global objects, for that matter) on higher AO levels is pointless and unlike for allocating individual objects, which may happen outside of regular JS execution, we don't need control over the specific realm that is being used there.
45 lines
1.2 KiB
C++
45 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2021, Idan Horowitz <idan.horowitz@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibJS/Runtime/GlobalObject.h>
|
|
#include <LibJS/Runtime/Map.h>
|
|
#include <LibJS/Runtime/Object.h>
|
|
#include <LibJS/Runtime/Value.h>
|
|
|
|
namespace JS {
|
|
|
|
class Set : public Object {
|
|
JS_OBJECT(Set, Object);
|
|
|
|
public:
|
|
static Set* create(Realm&);
|
|
|
|
explicit Set(Object& prototype);
|
|
virtual ~Set() override = default;
|
|
|
|
// NOTE: Unlike what the spec says, we implement Sets using an underlying map,
|
|
// so all the functions below do not directly implement the operations as
|
|
// defined by the specification.
|
|
|
|
void set_clear() { m_values.map_clear(); }
|
|
bool set_remove(Value const& value) { return m_values.map_remove(value); }
|
|
bool set_has(Value const& key) const { return m_values.map_has(key); }
|
|
void set_add(Value const& key) { m_values.map_set(key, js_undefined()); }
|
|
size_t set_size() const { return m_values.map_size(); }
|
|
|
|
auto begin() const { return m_values.begin(); }
|
|
auto begin() { return m_values.begin(); }
|
|
auto end() const { return m_values.end(); }
|
|
|
|
private:
|
|
virtual void visit_edges(Visitor& visitor) override;
|
|
|
|
Map m_values;
|
|
};
|
|
|
|
}
|