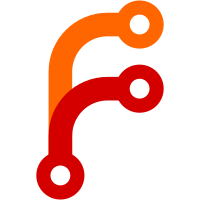
This patch adds function declaration hoisting. The mechanism is similar to var hoisting. Hoisted function declarations are to be put before the hoisted var declarations, hence they have to be treated separately.
37 lines
845 B
JavaScript
37 lines
845 B
JavaScript
load("test-common.js");
|
|
|
|
try {
|
|
var callHoisted = hoisted();
|
|
function hoisted() {
|
|
return true;
|
|
}
|
|
assert(hoisted() === true);
|
|
assert(callHoisted === true);
|
|
|
|
{
|
|
var callScopedHoisted = scopedHoisted();
|
|
function scopedHoisted() {
|
|
return "foo";
|
|
}
|
|
assert(scopedHoisted() === "foo");
|
|
assert(callScopedHoisted === "foo");
|
|
}
|
|
assert(scopedHoisted() === "foo");
|
|
assert(callScopedHoisted === "foo");
|
|
|
|
const test = () => {
|
|
var iife = (function () {
|
|
return declaredLater();
|
|
})();
|
|
function declaredLater() {
|
|
return "yay";
|
|
}
|
|
return iife;
|
|
};
|
|
assert(typeof declaredLater === "undefined");
|
|
assert(test() === "yay");
|
|
|
|
console.log("PASS");
|
|
} catch (e) {
|
|
console.log("FAIL: " + e);
|
|
}
|