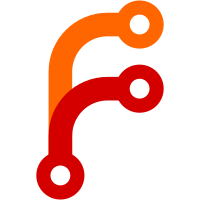
This patch makes it possible to call Node::invalidate_style() and have that node and all of its ancestors recompute their style. We then figure out if the new style is visually different from the old style, and if so do a paint invalidation with set_needs_display(). Note that the "are they visually different" code is very incomplete! Use this to make hover effects a lot more efficient. They no longer cause a full relayout+repaint, but only a style invalidation. Style invalidations are still quite heavy though, and there's a lot of room for improvement there. :^)
140 lines
4 KiB
C++
140 lines
4 KiB
C++
#include <LibHTML/CSS/StyleResolver.h>
|
|
#include <LibHTML/DOM/Document.h>
|
|
#include <LibHTML/DOM/Element.h>
|
|
#include <LibHTML/Layout/LayoutBlock.h>
|
|
#include <LibHTML/Layout/LayoutInline.h>
|
|
#include <LibHTML/Layout/LayoutListItem.h>
|
|
|
|
Element::Element(Document& document, const String& tag_name)
|
|
: ParentNode(document, NodeType::ELEMENT_NODE)
|
|
, m_tag_name(tag_name)
|
|
{
|
|
}
|
|
|
|
Element::~Element()
|
|
{
|
|
}
|
|
|
|
Attribute* Element::find_attribute(const String& name)
|
|
{
|
|
for (auto& attribute : m_attributes) {
|
|
if (attribute.name() == name)
|
|
return &attribute;
|
|
}
|
|
return nullptr;
|
|
}
|
|
|
|
const Attribute* Element::find_attribute(const String& name) const
|
|
{
|
|
for (auto& attribute : m_attributes) {
|
|
if (attribute.name() == name)
|
|
return &attribute;
|
|
}
|
|
return nullptr;
|
|
}
|
|
|
|
String Element::attribute(const String& name) const
|
|
{
|
|
if (auto* attribute = find_attribute(name))
|
|
return attribute->value();
|
|
return {};
|
|
}
|
|
|
|
void Element::set_attribute(const String& name, const String& value)
|
|
{
|
|
if (auto* attribute = find_attribute(name))
|
|
attribute->set_value(value);
|
|
else
|
|
m_attributes.empend(name, value);
|
|
|
|
parse_attribute(name, value);
|
|
}
|
|
|
|
void Element::set_attributes(Vector<Attribute>&& attributes)
|
|
{
|
|
m_attributes = move(attributes);
|
|
|
|
for (auto& attribute : m_attributes)
|
|
parse_attribute(attribute.name(), attribute.value());
|
|
}
|
|
|
|
bool Element::has_class(const StringView& class_name) const
|
|
{
|
|
auto value = attribute("class");
|
|
if (value.is_empty())
|
|
return false;
|
|
auto parts = value.split_view(' ');
|
|
for (auto& part : parts) {
|
|
if (part == class_name)
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
RefPtr<LayoutNode> Element::create_layout_node(const StyleResolver& resolver, const StyleProperties* parent_style) const
|
|
{
|
|
auto style = resolver.resolve_style(*this, parent_style);
|
|
|
|
auto display_property = style->property(CSS::PropertyID::Display);
|
|
String display = display_property.has_value() ? display_property.release_value()->to_string() : "inline";
|
|
|
|
if (display == "none")
|
|
return nullptr;
|
|
if (display == "block")
|
|
return adopt(*new LayoutBlock(this, move(style)));
|
|
if (display == "inline")
|
|
return adopt(*new LayoutInline(*this, move(style)));
|
|
if (display == "list-item")
|
|
return adopt(*new LayoutListItem(*this, move(style)));
|
|
|
|
ASSERT_NOT_REACHED();
|
|
}
|
|
|
|
void Element::parse_attribute(const String&, const String&)
|
|
{
|
|
}
|
|
|
|
enum class StyleDifference {
|
|
None,
|
|
NeedsRepaint,
|
|
NeedsRelayout,
|
|
};
|
|
|
|
static StyleDifference compute_style_difference(const StyleProperties& old_style, const StyleProperties& new_style, const Document& document)
|
|
{
|
|
if (old_style == new_style)
|
|
return StyleDifference::None;
|
|
|
|
bool needs_repaint = false;
|
|
bool needs_relayout = false;
|
|
|
|
if (new_style.color_or_fallback(CSS::PropertyID::Color, document, Color::Black) != old_style.color_or_fallback(CSS::PropertyID::Color, document, Color::Black))
|
|
needs_repaint = true;
|
|
else if (new_style.color_or_fallback(CSS::PropertyID::BackgroundColor, document, Color::Black) != old_style.color_or_fallback(CSS::PropertyID::BackgroundColor, document, Color::Black))
|
|
needs_repaint = true;
|
|
|
|
if (needs_relayout)
|
|
return StyleDifference::NeedsRelayout;
|
|
if (needs_repaint)
|
|
return StyleDifference::NeedsRepaint;
|
|
return StyleDifference::None;
|
|
}
|
|
|
|
void Element::recompute_style()
|
|
{
|
|
ASSERT(parent());
|
|
auto* parent_layout_node = parent()->layout_node();
|
|
ASSERT(parent_layout_node);
|
|
auto style = document().style_resolver().resolve_style(*this, &parent_layout_node->style());
|
|
ASSERT(layout_node());
|
|
auto diff = compute_style_difference(layout_node()->style(), *style, document());
|
|
if (diff == StyleDifference::None)
|
|
return;
|
|
layout_node()->set_style(*style);
|
|
if (diff == StyleDifference::NeedsRelayout) {
|
|
ASSERT_NOT_REACHED();
|
|
}
|
|
if (diff == StyleDifference::NeedsRepaint) {
|
|
layout_node()->set_needs_display();
|
|
}
|
|
}
|