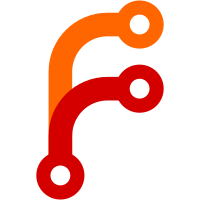
This is a prerequisite for upstreaming our LLVM patches, as our current hack forcing `-ftls-model=initial-exec` in the Clang driver is not acceptable upstream. Currently, our kernel-managed TLS implementation limits us to only having a single block of storage for all thread-local variables that's initialized at load time. This PR merely implements the dynamic TLS interface (`__tls_get_addr` and TLSDESC) on top of our static TLS infrastructure. The current model's limitations still stand: - a single static TLS block is reserved at load time, `dlopen()`-ing shared libraries that define thread-local variables might cause us to run out of space. - the initial TLS image is not changeable post-load, so `dlopen()`-ing libraries with non-zero-initialized TLS variables is not supported. The way we repurpose `ti_module` to mean "offset within static TLS block" instead of "module index" is not ABI-compliant.
39 lines
957 B
C
39 lines
957 B
C
/*
|
|
* Copyright (c) 2020-2023, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <sys/cdefs.h>
|
|
#include <sys/types.h>
|
|
|
|
__BEGIN_DECLS
|
|
|
|
typedef void (*AtExitFunction)(void*);
|
|
|
|
extern void __libc_init(void);
|
|
extern void __malloc_init(void);
|
|
extern void __stdio_init(void);
|
|
extern void __begin_atexit_locking(void);
|
|
extern void _init(void);
|
|
extern bool __environ_is_malloced;
|
|
extern bool __stdio_is_initialized;
|
|
extern bool __heap_is_stable;
|
|
extern void* __auxiliary_vector;
|
|
|
|
int __cxa_atexit(AtExitFunction exit_function, void* parameter, void* dso_handle);
|
|
void __cxa_finalize(void* dso_handle);
|
|
__attribute__((noreturn)) void __cxa_pure_virtual(void) __attribute__((weak));
|
|
__attribute__((noreturn)) void __stack_chk_fail(void);
|
|
__attribute__((noreturn)) void __stack_chk_fail_local(void);
|
|
|
|
struct __tls_index {
|
|
size_t ti_module;
|
|
size_t ti_offset;
|
|
};
|
|
|
|
void* __tls_get_addr(__tls_index*);
|
|
|
|
__END_DECLS
|