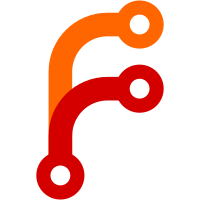
Our Reference class now has the same fields as the spec: - Base (a non-nullish value, an environment record, or `unresolvable`) - Referenced Name (the name of the binding) - Strict (whether the reference originated in strict mode code) - ThisValue (if non-empty, the reference represents a `super` keyword) The main difference from before is that we now resolve the environment record that a reference interacts with. Previously we simply resolved to either "local variable" or "global variable". The associated abstract operations are still largely non-conforming, since we don't yet implement proper variable bindings. But this patch should at least fix a handful of test262 cases. :^) There's one minor regression: some TypeError message strings get a little worse due to doing a RequireObjectCoercible earlier in the evaluation of MemberExpression.
26 lines
920 B
JavaScript
26 lines
920 B
JavaScript
"use strict";
|
|
|
|
test("basic functionality", () => {
|
|
[true, false, "foo", 123].forEach(primitive => {
|
|
expect(() => {
|
|
primitive.foo = "bar";
|
|
}).toThrowWithMessage(
|
|
TypeError,
|
|
`Cannot set property 'foo' of ${typeof primitive} '${primitive}'`
|
|
);
|
|
expect(() => {
|
|
primitive[Symbol.hasInstance] = 123;
|
|
}).toThrowWithMessage(
|
|
TypeError,
|
|
`Cannot set property 'Symbol(Symbol.hasInstance)' of ${typeof primitive} '${primitive}'`
|
|
);
|
|
});
|
|
[null, undefined].forEach(primitive => {
|
|
expect(() => {
|
|
primitive.foo = "bar";
|
|
}).toThrowWithMessage(TypeError, `${primitive} cannot be converted to an object`);
|
|
expect(() => {
|
|
primitive[Symbol.hasInstance] = 123;
|
|
}).toThrowWithMessage(TypeError, `${primitive} cannot be converted to an object`);
|
|
});
|
|
});
|