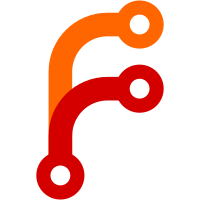
This introduces two new instructions: Jump and JumpIfFalse. Jumps are made to a Bytecode::Label, which is a simple object that represents a location in the bytecode stream. Note that you may not always know the target of a jump when adding the jump instruction itself, but we can just update the instruction later on during codegen once we know where the jump target is. The Bytecode::Interpreter now implements jumping via a jump slot that gets checked after each instruction to see if a jump is pending. If not, we just increment the PC as usual.
42 lines
790 B
C++
42 lines
790 B
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/OwnPtr.h>
|
|
#include <LibJS/Bytecode/Label.h>
|
|
#include <LibJS/Forward.h>
|
|
|
|
namespace JS::Bytecode {
|
|
|
|
class Generator {
|
|
public:
|
|
static OwnPtr<Block> generate(ASTNode const&);
|
|
|
|
Register allocate_register();
|
|
|
|
template<typename OpType, typename... Args>
|
|
OpType& emit(Args&&... args)
|
|
{
|
|
auto instruction = make<OpType>(forward<Args>(args)...);
|
|
auto* ptr = instruction.ptr();
|
|
append(move(instruction));
|
|
return *ptr;
|
|
}
|
|
|
|
Label make_label() const;
|
|
|
|
private:
|
|
Generator();
|
|
~Generator();
|
|
|
|
void append(NonnullOwnPtr<Instruction>);
|
|
|
|
OwnPtr<Block> m_block;
|
|
u32 m_next_register { 1 };
|
|
};
|
|
|
|
}
|