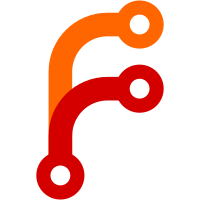
This introduces two new instructions: Jump and JumpIfFalse. Jumps are made to a Bytecode::Label, which is a simple object that represents a location in the bytecode stream. Note that you may not always know the target of a jump when adding the jump instruction itself, but we can just update the instruction later on during codegen once we know where the jump target is. The Bytecode::Interpreter now implements jumping via a jump slot that gets checked after each instruction to see if a jump is pending. If not, we just increment the PC as usual.
50 lines
1 KiB
C++
50 lines
1 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/OwnPtr.h>
|
|
#include <LibJS/AST.h>
|
|
#include <LibJS/Bytecode/Block.h>
|
|
#include <LibJS/Bytecode/Generator.h>
|
|
#include <LibJS/Bytecode/Instruction.h>
|
|
#include <LibJS/Bytecode/Register.h>
|
|
#include <LibJS/Forward.h>
|
|
|
|
namespace JS::Bytecode {
|
|
|
|
Generator::Generator()
|
|
{
|
|
m_block = Block::create();
|
|
}
|
|
|
|
Generator::~Generator()
|
|
{
|
|
}
|
|
|
|
OwnPtr<Block> Generator::generate(ASTNode const& node)
|
|
{
|
|
Generator generator;
|
|
[[maybe_unused]] auto dummy = node.generate_bytecode(generator);
|
|
generator.m_block->set_register_count({}, generator.m_next_register);
|
|
return move(generator.m_block);
|
|
}
|
|
|
|
void Generator::append(NonnullOwnPtr<Instruction> instruction)
|
|
{
|
|
m_block->append({}, move(instruction));
|
|
}
|
|
|
|
Register Generator::allocate_register()
|
|
{
|
|
VERIFY(m_next_register != NumericLimits<u32>::max());
|
|
return Register { m_next_register++ };
|
|
}
|
|
|
|
Label Generator::make_label() const
|
|
{
|
|
return Label { m_block->instructions().size() };
|
|
}
|
|
|
|
}
|