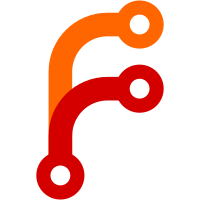
We now evaluate the conditions of `@media` rules at the same point in the HTML event loop as evaluation of `MediaQueryList`s. This is not strictly to spec, but since the spec doesn't actually say when to do this, it seems to make the most sense. In any case, it works! :^)
41 lines
1 KiB
C++
41 lines
1 KiB
C++
/*
|
|
* Copyright (c) 2021, Sam Atkins <atkinssj@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Function.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <LibWeb/CSS/CSSRule.h>
|
|
#include <LibWeb/CSS/CSSRuleList.h>
|
|
#include <LibWeb/Forward.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
class CSSGroupingRule : public CSSRule {
|
|
AK_MAKE_NONCOPYABLE(CSSGroupingRule);
|
|
AK_MAKE_NONMOVABLE(CSSGroupingRule);
|
|
|
|
public:
|
|
~CSSGroupingRule();
|
|
|
|
CSSRuleList const& css_rules() const { return m_rules; }
|
|
CSSRuleList& css_rules() { return m_rules; }
|
|
size_t insert_rule(StringView const& rule, size_t index = 0);
|
|
void delete_rule(size_t index);
|
|
|
|
virtual void for_each_effective_style_rule(Function<void(CSSStyleRule const&)> const& callback) const;
|
|
virtual bool for_first_not_loaded_import_rule(Function<void(CSSImportRule&)> const& callback);
|
|
|
|
virtual String serialized() const;
|
|
|
|
protected:
|
|
explicit CSSGroupingRule(NonnullRefPtrVector<CSSRule>&&);
|
|
|
|
private:
|
|
NonnullRefPtr<CSSRuleList> m_rules;
|
|
};
|
|
|
|
}
|