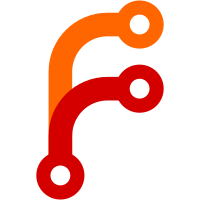
This function now takes an optional out parameter for callers who would like to what kind of property we ended up getting. This will be used to implement inline caching for property lookups. Also, to prepare for adding more forms of caching, the out parameter is a struct CacheablePropertyMetadata rather than just an offset. :^)
42 lines
1.3 KiB
C++
42 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibJS/Runtime/Completion.h>
|
|
#include <LibJS/Runtime/Environment.h>
|
|
#include <LibJS/Runtime/Object.h>
|
|
|
|
namespace JS {
|
|
|
|
class ArgumentsObject final : public Object {
|
|
JS_OBJECT(ArgumentsObject, Object);
|
|
|
|
public:
|
|
virtual ThrowCompletionOr<void> initialize(Realm&) override;
|
|
virtual ~ArgumentsObject() override = default;
|
|
|
|
Environment& environment() { return m_environment; }
|
|
|
|
virtual ThrowCompletionOr<Optional<PropertyDescriptor>> internal_get_own_property(PropertyKey const&) const override;
|
|
virtual ThrowCompletionOr<bool> internal_define_own_property(PropertyKey const&, PropertyDescriptor const&) override;
|
|
virtual ThrowCompletionOr<Value> internal_get(PropertyKey const&, Value receiver, CacheablePropertyMetadata*) const override;
|
|
virtual ThrowCompletionOr<bool> internal_set(PropertyKey const&, Value value, Value receiver) override;
|
|
virtual ThrowCompletionOr<bool> internal_delete(PropertyKey const&) override;
|
|
|
|
// [[ParameterMap]]
|
|
Object& parameter_map() { return *m_parameter_map; }
|
|
|
|
private:
|
|
ArgumentsObject(Realm&, Environment&);
|
|
|
|
virtual void visit_edges(Cell::Visitor&) override;
|
|
|
|
NonnullGCPtr<Environment> m_environment;
|
|
GCPtr<Object> m_parameter_map;
|
|
};
|
|
|
|
}
|