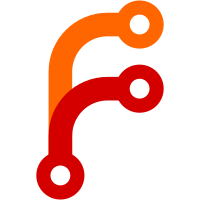
Since LayoutText inherits all of its style information from its parent Element anyway, it makes more sense to load the font at a higher level. And since the font depends only on the style and nothing else, this patch moves font loading (and caching) into StyleProperties. This could be made a lot smarter to avoid loading the same font many times, etc.
31 lines
897 B
C++
31 lines
897 B
C++
#pragma once
|
|
|
|
#include <LibHTML/DOM/Text.h>
|
|
#include <LibHTML/Layout/LayoutNode.h>
|
|
|
|
class LineBoxFragment;
|
|
|
|
class LayoutText : public LayoutNode {
|
|
public:
|
|
explicit LayoutText(const Text&);
|
|
virtual ~LayoutText() override;
|
|
|
|
const Text& node() const { return static_cast<const Text&>(*LayoutNode::node()); }
|
|
|
|
const String& text_for_style(const StyleProperties&) const;
|
|
|
|
virtual const char* class_name() const override { return "LayoutText"; }
|
|
virtual bool is_text() const final { return true; }
|
|
|
|
void render_fragment(RenderingContext&, const LineBoxFragment&) const;
|
|
|
|
virtual void split_into_lines(LayoutBlock& container) override;
|
|
|
|
const StyleProperties& style() const { return parent()->style(); }
|
|
|
|
private:
|
|
template<typename Callback>
|
|
void for_each_word(Callback) const;
|
|
template<typename Callback>
|
|
void for_each_source_line(Callback) const;
|
|
};
|