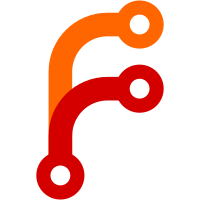
There was nothing left in ComputedStyle except the box model metrics, so this patch gives it a more representative name. Note that style information is fetched directly from StyleProperties, which is basically the CSS property name/value pairs that apply to an element.
32 lines
692 B
C++
32 lines
692 B
C++
#pragma once
|
|
|
|
#include <LibDraw/Size.h>
|
|
#include <LibHTML/CSS/LengthBox.h>
|
|
|
|
class BoxModelMetrics {
|
|
public:
|
|
BoxModelMetrics();
|
|
~BoxModelMetrics();
|
|
|
|
LengthBox& margin() { return m_margin; }
|
|
LengthBox& padding() { return m_padding; }
|
|
LengthBox& border() { return m_border; }
|
|
|
|
const LengthBox& margin() const { return m_margin; }
|
|
const LengthBox& padding() const { return m_padding; }
|
|
const LengthBox& border() const { return m_border; }
|
|
|
|
struct PixelBox {
|
|
int top;
|
|
int right;
|
|
int bottom;
|
|
int left;
|
|
};
|
|
|
|
PixelBox full_margin() const;
|
|
|
|
private:
|
|
LengthBox m_margin;
|
|
LengthBox m_padding;
|
|
LengthBox m_border;
|
|
};
|