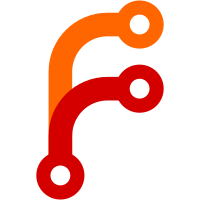
These are required for porting over Document from DeprecatedString to String. We unfortunately can't port this completely over yet as ParentNode is included by the Element IDL interface, which has not yet been ported over from DeprecatedString.
89 lines
2.8 KiB
C++
89 lines
2.8 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/DOM/Node.h>
|
|
|
|
namespace Web::DOM {
|
|
|
|
class ParentNode : public Node {
|
|
WEB_PLATFORM_OBJECT(ParentNode, Node);
|
|
|
|
public:
|
|
template<typename F>
|
|
void for_each_child(F) const;
|
|
template<typename F>
|
|
void for_each_child(F);
|
|
|
|
JS::GCPtr<Element> first_element_child();
|
|
JS::GCPtr<Element> last_element_child();
|
|
u32 child_element_count() const;
|
|
|
|
WebIDL::ExceptionOr<JS::GCPtr<Element>> query_selector(StringView);
|
|
WebIDL::ExceptionOr<JS::NonnullGCPtr<NodeList>> query_selector_all(StringView);
|
|
|
|
JS::NonnullGCPtr<HTMLCollection> children();
|
|
|
|
JS::NonnullGCPtr<HTMLCollection> get_elements_by_tag_name(FlyString const&);
|
|
JS::NonnullGCPtr<HTMLCollection> get_elements_by_tag_name_ns(Optional<String> const&, FlyString const&);
|
|
|
|
JS::NonnullGCPtr<HTMLCollection> get_elements_by_tag_name(DeprecatedFlyString const&);
|
|
JS::NonnullGCPtr<HTMLCollection> get_elements_by_tag_name_ns(DeprecatedFlyString const&, DeprecatedFlyString const&);
|
|
|
|
WebIDL::ExceptionOr<void> prepend(Vector<Variant<JS::Handle<Node>, String>> const& nodes);
|
|
WebIDL::ExceptionOr<void> append(Vector<Variant<JS::Handle<Node>, String>> const& nodes);
|
|
WebIDL::ExceptionOr<void> replace_children(Vector<Variant<JS::Handle<Node>, String>> const& nodes);
|
|
|
|
WebIDL::ExceptionOr<void> prepend(Vector<Variant<JS::Handle<Node>, DeprecatedString>> const& nodes);
|
|
WebIDL::ExceptionOr<void> append(Vector<Variant<JS::Handle<Node>, DeprecatedString>> const& nodes);
|
|
WebIDL::ExceptionOr<void> replace_children(Vector<Variant<JS::Handle<Node>, DeprecatedString>> const& nodes);
|
|
|
|
protected:
|
|
ParentNode(JS::Realm& realm, Document& document, NodeType type)
|
|
: Node(realm, document, type)
|
|
{
|
|
}
|
|
|
|
ParentNode(Document& document, NodeType type)
|
|
: Node(document, type)
|
|
{
|
|
}
|
|
|
|
virtual void visit_edges(Cell::Visitor&) override;
|
|
|
|
private:
|
|
JS::GCPtr<HTMLCollection> m_children;
|
|
};
|
|
|
|
template<>
|
|
inline bool Node::fast_is<ParentNode>() const { return is_parent_node(); }
|
|
|
|
template<typename U>
|
|
inline U* Node::shadow_including_first_ancestor_of_type()
|
|
{
|
|
for (auto* ancestor = parent_or_shadow_host(); ancestor; ancestor = ancestor->parent_or_shadow_host()) {
|
|
if (is<U>(*ancestor))
|
|
return &verify_cast<U>(*ancestor);
|
|
}
|
|
return nullptr;
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void ParentNode::for_each_child(Callback callback) const
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void ParentNode::for_each_child(Callback callback)
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
}
|