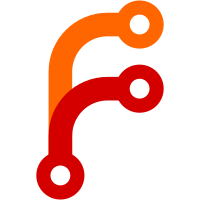
Using a special instruction to access global variables allows skipping the environment chain traversal for them and going directly to the module/global environment. Currently, this instruction only caches the offset for bindings that belong to the global object environment. However, there is also an opportunity to cache the offset in the global declarative record. This change results in a 57% increase in speed for imaging-gaussian-blur.js in Kraken.
44 lines
1.2 KiB
C++
44 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2021-2023, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/DeprecatedFlyString.h>
|
|
#include <AK/NonnullOwnPtr.h>
|
|
#include <AK/WeakPtr.h>
|
|
#include <LibJS/Bytecode/BasicBlock.h>
|
|
#include <LibJS/Bytecode/IdentifierTable.h>
|
|
#include <LibJS/Bytecode/StringTable.h>
|
|
|
|
namespace JS::Bytecode {
|
|
|
|
struct PropertyLookupCache {
|
|
WeakPtr<Shape> shape;
|
|
Optional<u32> property_offset;
|
|
u64 unique_shape_serial_number { 0 };
|
|
};
|
|
|
|
struct GlobalVariableCache : public PropertyLookupCache {
|
|
u64 environment_serial_number { 0 };
|
|
};
|
|
|
|
struct Executable {
|
|
DeprecatedFlyString name;
|
|
Vector<PropertyLookupCache> property_lookup_caches;
|
|
Vector<GlobalVariableCache> global_variable_caches;
|
|
Vector<NonnullOwnPtr<BasicBlock>> basic_blocks;
|
|
NonnullOwnPtr<StringTable> string_table;
|
|
NonnullOwnPtr<IdentifierTable> identifier_table;
|
|
size_t number_of_registers { 0 };
|
|
bool is_strict_mode { false };
|
|
|
|
DeprecatedString const& get_string(StringTableIndex index) const { return string_table->get(index); }
|
|
DeprecatedFlyString const& get_identifier(IdentifierTableIndex index) const { return identifier_table->get(index); }
|
|
|
|
void dump() const;
|
|
};
|
|
|
|
}
|