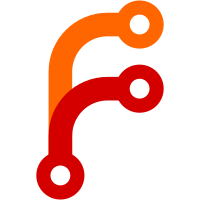
The support in LibWeb is quite easy as the previous commits introduced helpers to support lab-like colors. Now for the methods in Color: - The formulas in `from_lab()` are derived from the CIEXYZ to CIELAB formulas the "Colorimetry" paper published by the CIE. - The conversion in `from_xyz50()` can be decomposed in multiple steps XYZ D50 -> XYZ D65 -> Linear sRGB -> sRGB. The two first conversion are done with a singular matrix operation. This matrix was generated with a Python script [1]. This commit makes us pass all the `css/css-color/lab-00*.html` WPT tests (0 to 7 at the time of writing). [1] Python script used to generate the XYZ D50 -> Linear sRGB conversion: ```python import numpy as np # http://www.brucelindbloom.com/index.html?Eqn_ChromAdapt.html # First let's convert from D50 to D65 using the Bradford method. m_a = np.array([ [0.8951000, 0.2664000, -0.1614000], [-0.7502000, 1.7135000, 0.0367000], [0.0389000, -0.0685000, 1.0296000] ]) # D50 chromaticities_source = np.array([0.96422, 1, 0.82521]) # D65 chromaticities_destination = np.array([0.9505, 1, 1.0890]) cone_response_source = m_a @ chromaticities_source cone_response_destination = m_a @ chromaticities_destination cone_response_ratio = cone_response_destination / cone_response_source m = np.linalg.inv(m_a) @ np.diagflat(cone_response_ratio) @ m_a D50_to_D65 = m # https://en.wikipedia.org/wiki/SRGB#From_CIE_XYZ_to_sRGB # Then, the matrix to convert to linear sRGB. xyz_65_to_srgb = np.array([ [3.2406, - 1.5372, - 0.4986], [-0.9689, + 1.8758, 0.0415], [0.0557, - 0.2040, 1.0570] ]) # Finally, let's retrieve the final transformation. xyz_50_to_srgb = xyz_65_to_srgb @ D50_to_D65 print(xyz_50_to_srgb) ```
85 lines
3.4 KiB
C++
85 lines
3.4 KiB
C++
/*
|
|
* Copyright (c) 2024, Sam Atkins <sam@ladybird.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/CSS/StyleValues/CSSColorValue.h>
|
|
#include <LibWeb/CSS/StyleValues/NumberStyleValue.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
class CSSLabLike : public CSSColorValue {
|
|
public:
|
|
virtual ~CSSLabLike() override = default;
|
|
|
|
CSSStyleValue const& l() const { return *m_properties.l; }
|
|
CSSStyleValue const& a() const { return *m_properties.a; }
|
|
CSSStyleValue const& b() const { return *m_properties.b; }
|
|
CSSStyleValue const& alpha() const { return *m_properties.alpha; }
|
|
|
|
virtual bool equals(CSSStyleValue const& other) const override;
|
|
|
|
protected:
|
|
CSSLabLike(ColorType color_type, ValueComparingNonnullRefPtr<CSSStyleValue> l, ValueComparingNonnullRefPtr<CSSStyleValue> a, ValueComparingNonnullRefPtr<CSSStyleValue> b, ValueComparingNonnullRefPtr<CSSStyleValue> alpha)
|
|
: CSSColorValue(color_type)
|
|
, m_properties { .l = move(l), .a = move(a), .b = move(b), .alpha = move(alpha) }
|
|
{
|
|
}
|
|
|
|
struct Properties {
|
|
ValueComparingNonnullRefPtr<CSSStyleValue> l;
|
|
ValueComparingNonnullRefPtr<CSSStyleValue> a;
|
|
ValueComparingNonnullRefPtr<CSSStyleValue> b;
|
|
ValueComparingNonnullRefPtr<CSSStyleValue> alpha;
|
|
bool operator==(Properties const&) const = default;
|
|
} m_properties;
|
|
};
|
|
|
|
// https://drafts.css-houdini.org/css-typed-om-1/#cssoklab
|
|
class CSSOKLab final : public CSSLabLike {
|
|
public:
|
|
static ValueComparingNonnullRefPtr<CSSOKLab> create(ValueComparingNonnullRefPtr<CSSStyleValue> l, ValueComparingNonnullRefPtr<CSSStyleValue> a, ValueComparingNonnullRefPtr<CSSStyleValue> b, ValueComparingRefPtr<CSSStyleValue> alpha = {})
|
|
{
|
|
// alpha defaults to 1
|
|
if (!alpha)
|
|
return adopt_ref(*new (nothrow) CSSOKLab(move(l), move(a), move(b), NumberStyleValue::create(1)));
|
|
|
|
return adopt_ref(*new (nothrow) CSSOKLab(move(l), move(a), move(b), alpha.release_nonnull()));
|
|
}
|
|
|
|
virtual Color to_color(Optional<Layout::NodeWithStyle const&>) const override;
|
|
virtual String to_string() const override;
|
|
|
|
private:
|
|
CSSOKLab(ValueComparingNonnullRefPtr<CSSStyleValue> l, ValueComparingNonnullRefPtr<CSSStyleValue> a, ValueComparingNonnullRefPtr<CSSStyleValue> b, ValueComparingNonnullRefPtr<CSSStyleValue> alpha)
|
|
: CSSLabLike(ColorType::OKLab, move(l), move(a), move(b), move(alpha))
|
|
{
|
|
}
|
|
};
|
|
|
|
// https://drafts.css-houdini.org/css-typed-om-1/#csslab
|
|
class CSSLab final : public CSSLabLike {
|
|
public:
|
|
static ValueComparingNonnullRefPtr<CSSLab> create(ValueComparingNonnullRefPtr<CSSStyleValue> l, ValueComparingNonnullRefPtr<CSSStyleValue> a, ValueComparingNonnullRefPtr<CSSStyleValue> b, ValueComparingRefPtr<CSSStyleValue> alpha = {})
|
|
{
|
|
// alpha defaults to 1
|
|
if (!alpha)
|
|
return adopt_ref(*new (nothrow) CSSLab(move(l), move(a), move(b), NumberStyleValue::create(1)));
|
|
|
|
return adopt_ref(*new (nothrow) CSSLab(move(l), move(a), move(b), alpha.release_nonnull()));
|
|
}
|
|
|
|
virtual Color to_color(Optional<Layout::NodeWithStyle const&>) const override;
|
|
virtual String to_string() const override;
|
|
|
|
private:
|
|
CSSLab(ValueComparingNonnullRefPtr<CSSStyleValue> l, ValueComparingNonnullRefPtr<CSSStyleValue> a, ValueComparingNonnullRefPtr<CSSStyleValue> b, ValueComparingNonnullRefPtr<CSSStyleValue> alpha)
|
|
: CSSLabLike(ColorType::Lab, move(l), move(a), move(b), move(alpha))
|
|
{
|
|
}
|
|
};
|
|
|
|
}
|