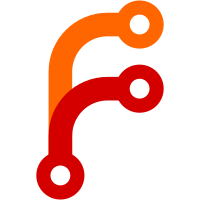
The support in LibWeb is quite easy as the previous commits introduced helpers to support lab-like colors. Now for the methods in Color: - The formulas in `from_lab()` are derived from the CIEXYZ to CIELAB formulas the "Colorimetry" paper published by the CIE. - The conversion in `from_xyz50()` can be decomposed in multiple steps XYZ D50 -> XYZ D65 -> Linear sRGB -> sRGB. The two first conversion are done with a singular matrix operation. This matrix was generated with a Python script [1]. This commit makes us pass all the `css/css-color/lab-00*.html` WPT tests (0 to 7 at the time of writing). [1] Python script used to generate the XYZ D50 -> Linear sRGB conversion: ```python import numpy as np # http://www.brucelindbloom.com/index.html?Eqn_ChromAdapt.html # First let's convert from D50 to D65 using the Bradford method. m_a = np.array([ [0.8951000, 0.2664000, -0.1614000], [-0.7502000, 1.7135000, 0.0367000], [0.0389000, -0.0685000, 1.0296000] ]) # D50 chromaticities_source = np.array([0.96422, 1, 0.82521]) # D65 chromaticities_destination = np.array([0.9505, 1, 1.0890]) cone_response_source = m_a @ chromaticities_source cone_response_destination = m_a @ chromaticities_destination cone_response_ratio = cone_response_destination / cone_response_source m = np.linalg.inv(m_a) @ np.diagflat(cone_response_ratio) @ m_a D50_to_D65 = m # https://en.wikipedia.org/wiki/SRGB#From_CIE_XYZ_to_sRGB # Then, the matrix to convert to linear sRGB. xyz_65_to_srgb = np.array([ [3.2406, - 1.5372, - 0.4986], [-0.9689, + 1.8758, 0.0415], [0.0557, - 0.2040, 1.0570] ]) # Finally, let's retrieve the final transformation. xyz_50_to_srgb = xyz_65_to_srgb @ D50_to_D65 print(xyz_50_to_srgb) ```
61 lines
2.1 KiB
C++
61 lines
2.1 KiB
C++
/*
|
|
* Copyright (c) 2024, Sam Atkins <sam@ladybird.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "CSSLabLike.h"
|
|
#include <AK/TypeCasts.h>
|
|
#include <LibWeb/CSS/Serialize.h>
|
|
#include <LibWeb/CSS/StyleValues/CSSMathValue.h>
|
|
#include <LibWeb/CSS/StyleValues/NumberStyleValue.h>
|
|
#include <LibWeb/CSS/StyleValues/PercentageStyleValue.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
bool CSSLabLike::equals(CSSStyleValue const& other) const
|
|
{
|
|
if (type() != other.type())
|
|
return false;
|
|
auto const& other_color = other.as_color();
|
|
if (color_type() != other_color.color_type())
|
|
return false;
|
|
auto const& other_lab_like = verify_cast<CSSLabLike>(other_color);
|
|
return m_properties == other_lab_like.m_properties;
|
|
}
|
|
|
|
Color CSSOKLab::to_color(Optional<Layout::NodeWithStyle const&>) const
|
|
{
|
|
auto const l_val = clamp(resolve_with_reference_value(m_properties.l, 1.0).value_or(0), 0, 1);
|
|
auto const a_val = resolve_with_reference_value(m_properties.a, 0.4).value_or(0);
|
|
auto const b_val = resolve_with_reference_value(m_properties.b, 0.4).value_or(0);
|
|
auto const alpha_val = resolve_alpha(m_properties.alpha).value_or(1);
|
|
|
|
return Color::from_oklab(l_val, a_val, b_val, alpha_val);
|
|
}
|
|
|
|
// https://www.w3.org/TR/css-color-4/#serializing-oklab-oklch
|
|
String CSSOKLab::to_string() const
|
|
{
|
|
// FIXME: Do this properly, taking unresolved calculated values into account.
|
|
return serialize_a_srgb_value(to_color({}));
|
|
}
|
|
|
|
Color CSSLab::to_color(Optional<Layout::NodeWithStyle const&>) const
|
|
{
|
|
auto const l_val = clamp(resolve_with_reference_value(m_properties.l, 100).value_or(0), 0, 100);
|
|
auto const a_val = resolve_with_reference_value(m_properties.a, 125).value_or(0);
|
|
auto const b_val = resolve_with_reference_value(m_properties.b, 125).value_or(0);
|
|
auto const alpha_val = resolve_alpha(m_properties.alpha).value_or(1);
|
|
|
|
return Color::from_lab(l_val, a_val, b_val, alpha_val);
|
|
}
|
|
|
|
// https://www.w3.org/TR/css-color-4/#serializing-lab-lch
|
|
String CSSLab::to_string() const
|
|
{
|
|
// FIXME: Do this properly, taking unresolved calculated values into account.
|
|
return serialize_a_srgb_value(to_color({}));
|
|
}
|
|
|
|
}
|