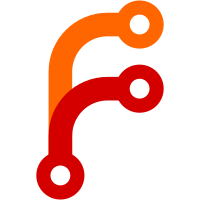
This removes a set of complex reference cycles between DOM, layout tree and browsing context. It also makes lifetimes much easier to reason about, as the DOM and layout trees are now free to keep each other alive.
36 lines
1.1 KiB
C++
36 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020, Matthew Olsson <mattco@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibWeb/Layout/SVGGraphicsBox.h>
|
|
#include <LibWeb/SVG/SVGGeometryElement.h>
|
|
|
|
namespace Web::Layout {
|
|
|
|
class SVGGeometryBox final : public SVGGraphicsBox {
|
|
JS_CELL(SVGGeometryBox, SVGGraphicsBox);
|
|
|
|
public:
|
|
SVGGeometryBox(DOM::Document&, SVG::SVGGeometryElement&, NonnullRefPtr<CSS::StyleProperties>);
|
|
virtual ~SVGGeometryBox() override = default;
|
|
|
|
SVG::SVGGeometryElement& dom_node() { return verify_cast<SVG::SVGGeometryElement>(SVGGraphicsBox::dom_node()); }
|
|
SVG::SVGGeometryElement const& dom_node() const { return verify_cast<SVG::SVGGeometryElement>(SVGGraphicsBox::dom_node()); }
|
|
|
|
float viewbox_scaling() const;
|
|
Gfx::FloatPoint viewbox_origin() const;
|
|
|
|
virtual RefPtr<Painting::Paintable> create_paintable() const override;
|
|
|
|
private:
|
|
virtual bool is_svg_geometry_box() const final { return true; }
|
|
};
|
|
|
|
template<>
|
|
inline bool Node::fast_is<SVGGeometryBox>() const { return is_svg_geometry_box(); }
|
|
|
|
}
|