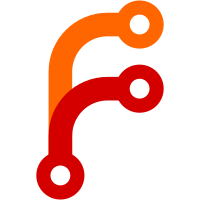
With this change, System::foo() becomes Core::System::foo(). Since LibCore builds on other systems than SerenityOS, we now have to make sure that wrappers work with just a standard C library underneath.
47 lines
1.5 KiB
C++
47 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibCore/System.h>
|
|
#include <LibSystem/syscall.h>
|
|
|
|
#define HANDLE_SYSCALL_RETURN_VALUE(syscall_name, rc, success_value) \
|
|
if ((rc) < 0) { \
|
|
return Error::from_syscall(syscall_name, rc); \
|
|
} \
|
|
return success_value;
|
|
|
|
namespace Core::System {
|
|
|
|
#ifdef __serenity__
|
|
ErrorOr<void> pledge(StringView promises, StringView execpromises)
|
|
{
|
|
Syscall::SC_pledge_params params {
|
|
{ promises.characters_without_null_termination(), promises.length() },
|
|
{ execpromises.characters_without_null_termination(), execpromises.length() },
|
|
};
|
|
int rc = syscall(SC_pledge, ¶ms);
|
|
HANDLE_SYSCALL_RETURN_VALUE("pledge"sv, rc, {});
|
|
}
|
|
|
|
ErrorOr<void> unveil(StringView path, StringView permissions)
|
|
{
|
|
Syscall::SC_unveil_params params {
|
|
{ path.characters_without_null_termination(), path.length() },
|
|
{ permissions.characters_without_null_termination(), permissions.length() },
|
|
};
|
|
int rc = syscall(SC_unveil, ¶ms);
|
|
HANDLE_SYSCALL_RETURN_VALUE("unveil"sv, rc, {});
|
|
}
|
|
#endif
|
|
|
|
ErrorOr<void> sigaction(int signal, struct sigaction const* action, struct sigaction* old_action)
|
|
{
|
|
if (::sigaction(signal, action, old_action) < 0)
|
|
return Error::from_syscall("sigaction"sv, -errno);
|
|
return {};
|
|
}
|
|
|
|
}
|