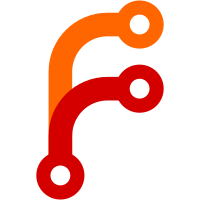
ACPI subsystem includes 3 types of parsers that are created during runtime, each one capable of parsing ACPI tables at different level. ACPIParser is the most basic parser which is essentialy a parser that can't parse anything useful, due to a user request to disable ACPI support in a kernel boot parameter. ACPIStaticParser is a derived class from ACPIParser, which is able to parse only static data (e.g. FADT, HPET, MCFG and other tables), thus making it not able to parse AML (ACPI Machine Language) nor to support handling of hardware events and power management. This type of parser can be created with a kernel boot parameter. ACPIDynamicParser is a derived class from ACPIStaticParser, which includes all the capabilities of the latter, but *should* implement an AML interpretation, (by building the ACPI AML namespace) and handling power & hardware events. Currently the methods to support AML interpretation are not implemented. This type of parser is created automatically during runtime if the user didn't specify a boot parameter related to ACPI initialization. Also, adding strncmp function definition in StdLib.h, to be able to use it in ACPIStaticParser class.
42 lines
No EOL
1.3 KiB
C++
42 lines
No EOL
1.3 KiB
C++
#pragma once
|
|
|
|
#include <ACPI/ACPIParser.h>
|
|
#include <AK/OwnPtr.h>
|
|
|
|
class ACPIStaticParser : ACPIParser {
|
|
public:
|
|
static void initialize(ACPI_RAW::RSDPDescriptor20& rsdp);
|
|
static void initialize_without_rsdp();
|
|
static bool is_initialized();
|
|
|
|
virtual ACPI_RAW::SDTHeader* find_table(const char* sig) override;
|
|
virtual void do_acpi_reboot() override;
|
|
virtual void do_acpi_shutdown() override;
|
|
virtual bool is_operable() override { return m_operable; }
|
|
|
|
protected:
|
|
ACPIStaticParser();
|
|
explicit ACPIStaticParser(ACPI_RAW::RSDPDescriptor20&);
|
|
|
|
virtual void mmap(VirtualAddress preferred_vaddr, PhysicalAddress paddr, u32) override;
|
|
virtual void mmap_region(Region& region, PhysicalAddress paddr) override;
|
|
|
|
private:
|
|
void locate_static_data();
|
|
void locate_all_aml_tables();
|
|
void locate_main_system_description_table();
|
|
void initialize_main_system_description_table();
|
|
ACPI_RAW::SDTHeader& find_fadt();
|
|
void init_fadt();
|
|
ACPI_RAW::RSDPDescriptor20* search_rsdp();
|
|
|
|
// Early pointers that are needed really for initializtion only...
|
|
ACPI_RAW::RSDPDescriptor20* m_rsdp;
|
|
ACPI_RAW::SDTHeader* m_main_system_description_table;
|
|
|
|
OwnPtr<ACPI::MainSystemDescriptionTable> m_main_sdt;
|
|
OwnPtr<ACPI::FixedACPIData> m_fadt;
|
|
|
|
Vector<ACPI_RAW::SDTHeader*> m_aml_tables_ptrs;
|
|
bool m_xsdt_supported;
|
|
}; |