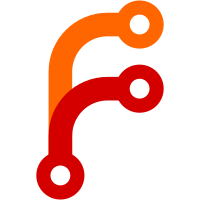
This is a normative change in the Intl.NumberFormat V3 spec. See: https://github.com/tc39/proposal-intl-numberformat-v3/commit/08f599b Note that this didn't seem to actually affect our implementation. The Unicode spec states: https://www.unicode.org/reports/tr35/tr35-53/tr35-numbers.html#Plural_Ranges "If there is no value for a <start,end> pair, the default result is end" Therefore, our implementation did not have the behavior noted by the issue this normative change addressed: const pr = new Intl.PluralRules("en-US"); pr.selectRange(1, 1); // Is "other", should be "one" Our implementation already returned "one" here because there is no such <start=one, end=one> value in the CLDR for en-US. Thus, we already returned the end value of "one".
46 lines
1.7 KiB
C++
46 lines
1.7 KiB
C++
/*
|
|
* Copyright (c) 2022-2023, Tim Flynn <trflynn89@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <AK/StringView.h>
|
|
#include <LibJS/Runtime/Completion.h>
|
|
#include <LibJS/Runtime/Intl/NumberFormat.h>
|
|
#include <LibJS/Runtime/Object.h>
|
|
#include <LibLocale/PluralRules.h>
|
|
|
|
namespace JS::Intl {
|
|
|
|
class PluralRules final : public NumberFormatBase {
|
|
JS_OBJECT(PluralRules, NumberFormatBase);
|
|
|
|
public:
|
|
virtual ~PluralRules() override = default;
|
|
|
|
::Locale::PluralForm type() const { return m_type; }
|
|
StringView type_string() const { return ::Locale::plural_form_to_string(m_type); }
|
|
void set_type(StringView type) { m_type = ::Locale::plural_form_from_string(type); }
|
|
|
|
private:
|
|
explicit PluralRules(Object& prototype);
|
|
|
|
::Locale::PluralForm m_type { ::Locale::PluralForm::Cardinal }; // [[Type]]
|
|
};
|
|
|
|
struct ResolvedPlurality {
|
|
::Locale::PluralCategory plural_category; // [[PluralCategory]]
|
|
String formatted_string; // [[FormattedString]]
|
|
};
|
|
|
|
::Locale::PluralOperands get_operands(StringView string);
|
|
::Locale::PluralCategory plural_rule_select(StringView locale, ::Locale::PluralForm type, Value number, ::Locale::PluralOperands operands);
|
|
ThrowCompletionOr<ResolvedPlurality> resolve_plural(VM&, PluralRules const&, Value number);
|
|
ThrowCompletionOr<ResolvedPlurality> resolve_plural(VM&, NumberFormatBase const& number_format, ::Locale::PluralForm type, Value number);
|
|
::Locale::PluralCategory plural_rule_select_range(StringView locale, ::Locale::PluralForm, ::Locale::PluralCategory start, ::Locale::PluralCategory end);
|
|
ThrowCompletionOr<::Locale::PluralCategory> resolve_plural_range(VM&, PluralRules const&, Value start, Value end);
|
|
|
|
}
|