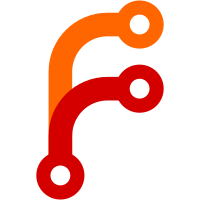
Previously: CSSImportRule::loaded_style_sheet() (and others) depend on the definition of class CSSStyleSheet. Meanwhile, CSSStyleSheet::template for_each_effective_style_rule (and others) depend on the definition of class CSSImportRule. This hasn't caused any problems so far because CSSStyleSheet.h happened to be always included after CSSImportRule.h (in part due to alphabetical ordering). However, a compilation unit that (for example) only contains #include <Userland/Libraries/LibWeb/CSSImportRule.h> would fail to compile. This patch resolves this issue by pushing the inline definition of Web::CSS::CSSStyleSheet::for_each_effective_style_rule and for_first_not_loaded_import_rule into a different file, and adding the missing headers.
91 lines
3.1 KiB
C++
91 lines
3.1 KiB
C++
/*
|
|
* Copyright (c) 2019-2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibWeb/CSS/CSSImportRule.h>
|
|
#include <LibWeb/CSS/CSSStyleSheet.h>
|
|
#include <LibWeb/CSS/Parser/Parser.h>
|
|
#include <LibWeb/DOM/ExceptionOr.h>
|
|
|
|
namespace Web::CSS {
|
|
|
|
CSSStyleSheet::CSSStyleSheet(NonnullRefPtrVector<CSSRule> rules)
|
|
: m_rules(CSSRuleList::create(move(rules)))
|
|
{
|
|
}
|
|
|
|
CSSStyleSheet::~CSSStyleSheet()
|
|
{
|
|
}
|
|
|
|
// https://drafts.csswg.org/cssom/#dom-cssstylesheet-insertrule
|
|
DOM::ExceptionOr<unsigned> CSSStyleSheet::insert_rule(StringView rule, unsigned index)
|
|
{
|
|
// FIXME: 1. If the origin-clean flag is unset, throw a SecurityError exception.
|
|
|
|
// FIXME: 2. If the disallow modification flag is set, throw a NotAllowedError DOMException.
|
|
|
|
// 3. Let parsed rule be the return value of invoking parse a rule with rule.
|
|
auto parsed_rule = parse_css_rule(CSS::ParsingContext {}, rule);
|
|
|
|
// 4. If parsed rule is a syntax error, return parsed rule.
|
|
if (!parsed_rule)
|
|
return DOM::SyntaxError::create("Unable to parse CSS rule.");
|
|
|
|
// FIXME: 5. If parsed rule is an @import rule, and the constructed flag is set, throw a SyntaxError DOMException.
|
|
|
|
// 6. Return the result of invoking insert a CSS rule rule in the CSS rules at index.
|
|
return m_rules->insert_a_css_rule(parsed_rule.release_nonnull(), index);
|
|
}
|
|
|
|
// https://drafts.csswg.org/cssom/#dom-cssstylesheet-deleterule
|
|
DOM::ExceptionOr<void> CSSStyleSheet::delete_rule(unsigned index)
|
|
{
|
|
// FIXME: 1. If the origin-clean flag is unset, throw a SecurityError exception.
|
|
|
|
// FIXME: 2. If the disallow modification flag is set, throw a NotAllowedError DOMException.
|
|
|
|
// 3. Remove a CSS rule in the CSS rules at index.
|
|
return m_rules->remove_a_css_rule(index);
|
|
}
|
|
|
|
// https://drafts.csswg.org/cssom/#dom-cssstylesheet-removerule
|
|
DOM::ExceptionOr<void> CSSStyleSheet::remove_rule(unsigned index)
|
|
{
|
|
// The removeRule(index) method must run the same steps as deleteRule().
|
|
return delete_rule(index);
|
|
}
|
|
|
|
void CSSStyleSheet::for_each_effective_style_rule(Function<void(CSSStyleRule const&)> const& callback) const
|
|
{
|
|
for (auto& rule : *m_rules)
|
|
if (rule.type() == CSSRule::Type::Style) {
|
|
callback(verify_cast<CSSStyleRule>(rule));
|
|
} else if (rule.type() == CSSRule::Type::Import) {
|
|
const auto& import_rule = verify_cast<CSSImportRule>(rule);
|
|
if (import_rule.has_import_result())
|
|
import_rule.loaded_style_sheet()->for_each_effective_style_rule(callback);
|
|
}
|
|
}
|
|
|
|
bool CSSStyleSheet::for_first_not_loaded_import_rule(Function<void(CSSImportRule&)> const& callback)
|
|
{
|
|
for (auto& rule : *m_rules)
|
|
if (rule.type() == CSSRule::Type::Import) {
|
|
auto& import_rule = verify_cast<CSSImportRule>(rule);
|
|
if (!import_rule.has_import_result()) {
|
|
callback(import_rule);
|
|
return true;
|
|
}
|
|
|
|
if (import_rule.loaded_style_sheet()->for_first_not_loaded_import_rule(callback)) {
|
|
return true;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
}
|