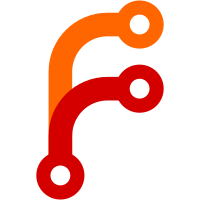
This limits the size of each block (currently set to 1K), and gets us closer to a canonical, more easily analysable bytecode format. As a result of this, "Labels" are now simply entries to basic blocks. Since there is no more 'conditional' jump (as all jumps are always taken), JumpIf{True,False} are unified to JumpConditional, and JumpIfNullish is renamed to JumpNullish. Also fixes #7914 as a result of reimplementing the loop logic.
65 lines
1.3 KiB
C++
65 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibJS/AST.h>
|
|
#include <LibJS/Bytecode/BasicBlock.h>
|
|
#include <LibJS/Bytecode/Generator.h>
|
|
#include <LibJS/Bytecode/Instruction.h>
|
|
#include <LibJS/Bytecode/Register.h>
|
|
#include <LibJS/Forward.h>
|
|
|
|
namespace JS::Bytecode {
|
|
|
|
Generator::Generator()
|
|
{
|
|
}
|
|
|
|
Generator::~Generator()
|
|
{
|
|
}
|
|
|
|
ExecutionUnit Generator::generate(ASTNode const& node)
|
|
{
|
|
Generator generator;
|
|
generator.switch_to_basic_block(generator.make_block());
|
|
node.generate_bytecode(generator);
|
|
return { move(generator.m_root_basic_blocks), generator.m_next_register };
|
|
}
|
|
|
|
void Generator::grow(size_t additional_size)
|
|
{
|
|
VERIFY(m_current_basic_block);
|
|
m_current_basic_block->grow(additional_size);
|
|
}
|
|
|
|
void* Generator::next_slot()
|
|
{
|
|
VERIFY(m_current_basic_block);
|
|
return m_current_basic_block->next_slot();
|
|
}
|
|
|
|
Register Generator::allocate_register()
|
|
{
|
|
VERIFY(m_next_register != NumericLimits<u32>::max());
|
|
return Register { m_next_register++ };
|
|
}
|
|
|
|
Label Generator::nearest_continuable_scope() const
|
|
{
|
|
return m_continuable_scopes.last();
|
|
}
|
|
|
|
void Generator::begin_continuable_scope(Label continue_target)
|
|
{
|
|
m_continuable_scopes.append(continue_target);
|
|
}
|
|
|
|
void Generator::end_continuable_scope()
|
|
{
|
|
m_continuable_scopes.take_last();
|
|
}
|
|
|
|
}
|