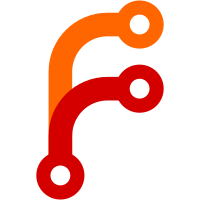
This is a very bulky way of doing this, and doesn't seem sustainable to implement every shorthand property this way, but it's a place to start. The "margin" CSS property now expands into its four longhands as far as my understanding of the specs. Note that shorthand expansion happens when we *resolve* style, not when we parse CSS. I'm not sure this is correct anymore, I think other UA's may actually expand shorthands into the declaration directly at parse these days. If so, we should do this at parsing as well.
47 lines
874 B
C++
47 lines
874 B
C++
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
|
|
class Length {
|
|
public:
|
|
enum class Type {
|
|
Auto,
|
|
Absolute,
|
|
};
|
|
|
|
Length() {}
|
|
Length(int value, Type type)
|
|
: m_type(type)
|
|
, m_value(value)
|
|
{
|
|
}
|
|
~Length() {}
|
|
|
|
bool is_auto() const { return m_type == Type::Auto; }
|
|
bool is_absolute() const { return m_type == Type::Absolute; }
|
|
|
|
int value() const { return m_value; }
|
|
|
|
String to_string() const
|
|
{
|
|
if (is_auto())
|
|
return "[Length/auto]";
|
|
return String::format("%d [Length/px]", m_value);
|
|
}
|
|
|
|
int to_px() const
|
|
{
|
|
if (is_auto())
|
|
return 0;
|
|
return m_value;
|
|
}
|
|
|
|
private:
|
|
Type m_type { Type::Auto };
|
|
int m_value { 0 };
|
|
};
|
|
|
|
inline const LogStream& operator<<(const LogStream& stream, const Length& value)
|
|
{
|
|
return stream << value.to_string();
|
|
}
|