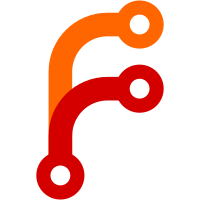
- Fixes No originally selected custom parameters in topic's edit tab #1534 - Adds test case for CustomParams to verify fix - Updates CustomParamsField to use predefined value - Renames INDEX_PREFIX to TOPIC_CUSTOM_PARAMS_PREFIX and moves it to constants file - Removes unused configs from Topic/Edit component - Rewrites DangerZone styled's - Rewrites DangerZone tests - Adds margin to DangerZone to match TopicForm width - Adds simple Topic/Edit test - Changes sonar-project.properties file's sonar.exclusions to correctly ignore paths Signed-off-by: Roman Zabaluev <rzabaluev@provectus.com> Co-authored-by: Roman Zabaluev <rzabaluev@provectus.com>
69 lines
1.8 KiB
TypeScript
69 lines
1.8 KiB
TypeScript
import React from 'react';
|
|
import { TopicFormData } from 'redux/interfaces';
|
|
import { useFieldArray, useFormContext, useWatch } from 'react-hook-form';
|
|
import { Button } from 'components/common/Button/Button';
|
|
import { TOPIC_CUSTOM_PARAMS_PREFIX } from 'lib/constants';
|
|
|
|
import CustomParamField from './CustomParamField';
|
|
import * as S from './CustomParams.styled';
|
|
|
|
export interface CustomParamsProps {
|
|
isSubmitting: boolean;
|
|
}
|
|
|
|
const CustomParams: React.FC<CustomParamsProps> = ({ isSubmitting }) => {
|
|
const { control } = useFormContext<TopicFormData>();
|
|
const { fields, append, remove } = useFieldArray({
|
|
control,
|
|
name: TOPIC_CUSTOM_PARAMS_PREFIX,
|
|
});
|
|
const watchFieldArray = useWatch({
|
|
control,
|
|
name: TOPIC_CUSTOM_PARAMS_PREFIX,
|
|
defaultValue: fields,
|
|
});
|
|
const controlledFields = fields.map((field, index) => {
|
|
return {
|
|
...field,
|
|
...watchFieldArray[index],
|
|
};
|
|
});
|
|
|
|
const [existingFields, setExistingFields] = React.useState<string[]>([]);
|
|
|
|
const removeField = (index: number): void => {
|
|
setExistingFields(
|
|
existingFields.filter((field) => field !== controlledFields[index].name)
|
|
);
|
|
remove(index);
|
|
};
|
|
|
|
return (
|
|
<S.ParamsWrapper>
|
|
{controlledFields.map((field, idx) => (
|
|
<CustomParamField
|
|
key={field.id}
|
|
field={field}
|
|
remove={removeField}
|
|
index={idx}
|
|
isDisabled={isSubmitting}
|
|
existingFields={existingFields}
|
|
setExistingFields={setExistingFields}
|
|
/>
|
|
))}
|
|
<div>
|
|
<Button
|
|
type="button"
|
|
buttonSize="M"
|
|
buttonType="secondary"
|
|
onClick={() => append({ name: '', value: '' })}
|
|
>
|
|
<i className="fas fa-plus" />
|
|
Add Custom Parameter
|
|
</Button>
|
|
</div>
|
|
</S.ParamsWrapper>
|
|
);
|
|
};
|
|
|
|
export default CustomParams;
|