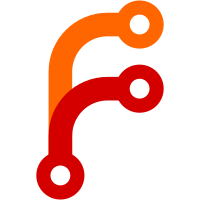
* Removed two enzyme test with testing-library tests * Got rid of enzyme and wrapper in List.spec.tsx * Got rid of enzyme and wrapper in ListItem.spec.tsx * Got rid of enzyme and wrapper in BytesFormatted.spec.tsx * Got rid of enzyme and wrapper in ConfirmationModal.spec.tsx * Got rid of enzyme and wrapper in Tabs.spec.tsx * Got rid of enzyme and wrapper in Actions.spec.tsx * Got rid of enzyme and wrapper in ListItem.spec.tsx * Got rid of enzyme and wrapper in FiltersContainer.spec.tsx * Got rid of enzyme and wrapper in ListItem.spec.tsx * Got rid of Enzyme in a two more files * Got rid of Enzyme in testHelpers.tsx * Got rid of snapshots * Three wrappers replaced with render from testHelpers * Testing id replaced * Fixed linter warnings * Got rid of testIds * Got rid of unnecessary containers and ...queryBy functions * Got rid of dublicated ...getByRole functions * Got rid of dublicated more than two times ...getByText functions * Got rid of unused imports * Got rid of unused import * Desciptions fixed * Got rid of providers * Got rid of unused imports * package-lock.json reverted * Refactor Actions component specs * Get rid of TestRouterWrapper * Refactor specs * Refactor specs * linting Co-authored-by: k.morozov <k.morozov@ffin.ru> Co-authored-by: lazzy-panda <grifx.design@gmail.com>
64 lines
1.5 KiB
TypeScript
64 lines
1.5 KiB
TypeScript
import React from 'react';
|
|
import { Button } from 'components/common/Button/Button';
|
|
|
|
import { ConfirmationModalWrapper } from './ConfirmationModal.styled';
|
|
|
|
export interface ConfirmationModalProps {
|
|
isOpen?: boolean;
|
|
title?: React.ReactNode;
|
|
onConfirm(): void;
|
|
onCancel(): void;
|
|
isConfirming?: boolean;
|
|
submitBtnText?: string;
|
|
}
|
|
|
|
const ConfirmationModal: React.FC<ConfirmationModalProps> = ({
|
|
isOpen,
|
|
children,
|
|
title = 'Confirm the action',
|
|
onCancel,
|
|
onConfirm,
|
|
isConfirming = false,
|
|
submitBtnText = 'Submit',
|
|
}) => {
|
|
const cancelHandler = React.useCallback(() => {
|
|
if (!isConfirming) {
|
|
onCancel();
|
|
}
|
|
}, [isConfirming, onCancel]);
|
|
|
|
return isOpen ? (
|
|
<ConfirmationModalWrapper>
|
|
<div onClick={cancelHandler} aria-hidden="true" role="button" />
|
|
<div>
|
|
<header>
|
|
<p>{title}</p>
|
|
</header>
|
|
<section>{children}</section>
|
|
<footer>
|
|
<Button
|
|
buttonType="secondary"
|
|
buttonSize="M"
|
|
onClick={cancelHandler}
|
|
type="button"
|
|
disabled={isConfirming}
|
|
>
|
|
Cancel
|
|
</Button>
|
|
|
|
<Button
|
|
buttonType="primary"
|
|
buttonSize="M"
|
|
onClick={onConfirm}
|
|
type="button"
|
|
disabled={isConfirming}
|
|
>
|
|
{submitBtnText}
|
|
</Button>
|
|
</footer>
|
|
</div>
|
|
</ConfirmationModalWrapper>
|
|
) : null;
|
|
};
|
|
|
|
export default ConfirmationModal;
|