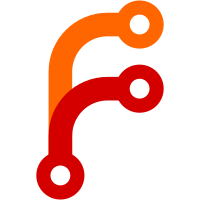
* got rid of react-hooks/exhaustive-deps errors - part 1 * got rid of react-hooks/exhaustive-deps errors in useSearch * got rid of react-hooks/exhaustive-deps errors in Filters * got rid of react-hooks/exhaustive-deps errors in ResetOffsets * got rid of react-hooks/exhaustive-deps errors in Filters * got rid of react-hooks/exhaustive-deps errors in Breadcrumbs * got rid of react-hooks/exhaustive-deps errors in DynamicTextButton * got rid of react-hooks/exhaustive-deps errors in useDataSaver * got rid of react-hooks/exhaustive-deps errors in ResultRenderer Co-authored-by: Roman Zabaluev <rzabaluev@provectus.com>
39 lines
1.1 KiB
TypeScript
39 lines
1.1 KiB
TypeScript
import useOutsideClickRef from '@rooks/use-outside-click-ref';
|
|
import cx from 'classnames';
|
|
import React, { useCallback, useMemo, useState } from 'react';
|
|
|
|
import * as S from './Dropdown.styled';
|
|
|
|
export interface DropdownProps {
|
|
label: React.ReactNode;
|
|
right?: boolean;
|
|
up?: boolean;
|
|
}
|
|
|
|
const Dropdown: React.FC<DropdownProps> = ({ label, right, up, children }) => {
|
|
const [active, setActive] = useState<boolean>(false);
|
|
const [wrapperRef] = useOutsideClickRef(() => setActive(false));
|
|
const onClick = useCallback(() => setActive(!active), [active]);
|
|
|
|
const classNames = useMemo(
|
|
() =>
|
|
cx('dropdown', {
|
|
'is-active': active,
|
|
'is-right': right,
|
|
'is-up': up,
|
|
}),
|
|
[active, right, up]
|
|
);
|
|
return (
|
|
<div className={classNames} ref={wrapperRef}>
|
|
<S.TriggerWrapper>
|
|
<S.Trigger onClick={onClick}>{label}</S.Trigger>
|
|
</S.TriggerWrapper>
|
|
<div className="dropdown-menu" id="dropdown-menu" role="menu">
|
|
<div className="dropdown-content has-text-left">{children}</div>
|
|
</div>
|
|
</div>
|
|
);
|
|
};
|
|
|
|
export default Dropdown;
|