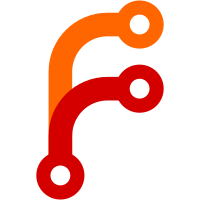
* got rid of react-hooks/exhaustive-deps errors - part 1 * got rid of react-hooks/exhaustive-deps errors in useSearch * got rid of react-hooks/exhaustive-deps errors in Filters * got rid of react-hooks/exhaustive-deps errors in ResetOffsets * got rid of react-hooks/exhaustive-deps errors in Filters * got rid of react-hooks/exhaustive-deps errors in Breadcrumbs * got rid of react-hooks/exhaustive-deps errors in DynamicTextButton * got rid of react-hooks/exhaustive-deps errors in useDataSaver * got rid of react-hooks/exhaustive-deps errors in ResultRenderer Co-authored-by: Roman Zabaluev <rzabaluev@provectus.com>
50 lines
1.2 KiB
TypeScript
50 lines
1.2 KiB
TypeScript
import React from 'react';
|
|
import { dismissAlert } from 'redux/actions';
|
|
import { getAlerts } from 'redux/reducers/alerts/selectors';
|
|
import { alertDissmissed, selectAll } from 'redux/reducers/alerts/alertsSlice';
|
|
import { useAppSelector, useAppDispatch } from 'lib/hooks/redux';
|
|
import Alert from 'components/Alerts/Alert';
|
|
|
|
const Alerts: React.FC = () => {
|
|
const alerts = useAppSelector(selectAll);
|
|
const dispatch = useAppDispatch();
|
|
const dismiss = React.useCallback(
|
|
(id: string) => {
|
|
dispatch(alertDissmissed(id));
|
|
},
|
|
[dispatch]
|
|
);
|
|
|
|
const legacyAlerts = useAppSelector(getAlerts);
|
|
const dismissLegacy = React.useCallback(
|
|
(id: string) => {
|
|
dispatch(dismissAlert(id));
|
|
},
|
|
[dispatch]
|
|
);
|
|
|
|
return (
|
|
<>
|
|
{alerts.map(({ id, type, title, message }) => (
|
|
<Alert
|
|
key={id}
|
|
type={type}
|
|
title={title}
|
|
message={message}
|
|
onDissmiss={() => dismiss(id)}
|
|
/>
|
|
))}
|
|
{legacyAlerts.map(({ id, type, title, message }) => (
|
|
<Alert
|
|
key={id}
|
|
type={type}
|
|
title={title}
|
|
message={message}
|
|
onDissmiss={() => dismissLegacy(id)}
|
|
/>
|
|
))}
|
|
</>
|
|
);
|
|
};
|
|
|
|
export default Alerts;
|