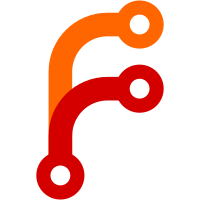
* remove withRouter HOC from FiltersContainer * remove withRouter HOC from Topics DetailsContainer * remove withRouter HOC from Topics TopicsConsumerGroupsContainer * withRouter HOC from Topics TopicsConsumerGroupsContainer * minor code refactor in the Details spec * Routes code modifications to refactor strings representation to functions * Settings and TopicsConsumer removal of HOC with Router * Remove withRouter HOC from Overview file * Remove withRouter HOC from Edit file * replace Router path with functions instead of strings * delete CustomParamsContainer and use the simple component in the TopicForm * remove HOC from DangerZone container * Remove withRouter HOC from Connect pages like Config , Overview , Tasks * Remove withRouter HOC from Connect pages like Actions, Details, Edit, New * Refactor Kafka Connect Codes * Refactor Topics pages * Remove HOC from Diff component and minor code refactor * Route component migration into children instead of renderProps or component param in App Component * Route component migration into children instead of renderProps or component param in Cluster Component * Route component migration into children instead of renderProps or component param in Topics Component * Route component migration into children instead of renderProps or component param in Topic Component * Route component migration into children instead of renderProps or component param in Topic Component * minor bug fix in the Overview selector spread * change Router from component Render to child render in ConsumerGroups page * change Router from component Render to child render in Schemas page * change Router from component Render to child render in KsqlDb page * change Router from component Render to child render in Connect page * change Router from component Render to child render in Connect Details page * Overview Details styling code modifications * All written path to paths with functions * Route Parameters code fix with functions and params with variables * Updating BreadCrumb Route * Refactor Redirects * WIP React Router v6 migration * Remove unused imports from the file * Make KsqlDb pages work with relative Routes * WIP Make Connect pages work and fix the Schema page testing problem * transforming consumer groups into relative path router * Transform Topics pages into relative routes * Transform Topic pages into relative routes * Minor changes in Connect and KsqlDb test suites relative routes * Minor changes in Connect and KsqlDb test suites relative routes * change the Details into relative Routes * Topics List naviagtion and caching issue fixed in tests suites * Topic New Naviagation issue fix + tests suites * Details navigate migrating into relative paths * Send Message Submit Naviagttion with tests suites * Topic Edit pages with working routes navigation * Topic Details and ResetOffsets Pages tests suites and navigations * Messages Table Tests suites * BreadCrumbs Routes fixes * ClusterMenu and Links styling minor code modifications * ClusterMenu and Links styling minor code modifications * Minor Code modifications * Fix Lintter Problems * fix Code Smells * create custom useParams hook * Adding Path tests * minor code refactors * Fix the Button Component redundant Props + transforming routes to relative * Fix linter issues
168 lines
5.9 KiB
TypeScript
168 lines
5.9 KiB
TypeScript
import React from 'react';
|
|
import { Partition, Replica } from 'generated-sources';
|
|
import { ClusterName, TopicName } from 'redux/interfaces';
|
|
import Dropdown from 'components/common/Dropdown/Dropdown';
|
|
import DropdownItem from 'components/common/Dropdown/DropdownItem';
|
|
import ClusterContext from 'components/contexts/ClusterContext';
|
|
import BytesFormatted from 'components/common/BytesFormatted/BytesFormatted';
|
|
import { Table } from 'components/common/table/Table/Table.styled';
|
|
import TableHeaderCell from 'components/common/table/TableHeaderCell/TableHeaderCell';
|
|
import VerticalElipsisIcon from 'components/common/Icons/VerticalElipsisIcon';
|
|
import * as Metrics from 'components/common/Metrics';
|
|
import { Tag } from 'components/common/Tag/Tag.styled';
|
|
import { useAppSelector } from 'lib/hooks/redux';
|
|
import { getTopicByName } from 'redux/reducers/topics/selectors';
|
|
import { ReplicaCell } from 'components/Topics/Topic/Details/Details.styled';
|
|
import { RouteParamsClusterTopic } from 'lib/paths';
|
|
import useAppParams from 'lib/hooks/useAppParams';
|
|
|
|
export interface Props {
|
|
clearTopicMessages(params: {
|
|
clusterName: ClusterName;
|
|
topicName: TopicName;
|
|
partitions?: number[];
|
|
}): void;
|
|
}
|
|
|
|
const Overview: React.FC<Props> = ({ clearTopicMessages }) => {
|
|
const { clusterName, topicName } = useAppParams<RouteParamsClusterTopic>();
|
|
|
|
const {
|
|
partitions,
|
|
underReplicatedPartitions,
|
|
inSyncReplicas,
|
|
replicas,
|
|
partitionCount,
|
|
internal,
|
|
replicationFactor,
|
|
segmentSize,
|
|
segmentCount,
|
|
cleanUpPolicy,
|
|
} = useAppSelector((state) => {
|
|
const res = getTopicByName(state, topicName);
|
|
return res || {};
|
|
});
|
|
|
|
const { isReadOnly } = React.useContext(ClusterContext);
|
|
|
|
const messageCount = React.useMemo(
|
|
() =>
|
|
(partitions || []).reduce((memo, partition) => {
|
|
return memo + partition.offsetMax - partition.offsetMin;
|
|
}, 0),
|
|
[partitions]
|
|
);
|
|
|
|
return (
|
|
<>
|
|
<Metrics.Wrapper>
|
|
<Metrics.Section>
|
|
<Metrics.Indicator label="Partitions">
|
|
{partitionCount}
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Replication Factor">
|
|
{replicationFactor}
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator
|
|
label="URP"
|
|
title="Under replicated partitions"
|
|
isAlert
|
|
alertType={underReplicatedPartitions === 0 ? 'success' : 'error'}
|
|
>
|
|
{underReplicatedPartitions === 0 ? (
|
|
<Metrics.LightText>{underReplicatedPartitions}</Metrics.LightText>
|
|
) : (
|
|
<Metrics.RedText>{underReplicatedPartitions}</Metrics.RedText>
|
|
)}
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator
|
|
label="In Sync Replicas"
|
|
isAlert
|
|
alertType={inSyncReplicas === replicas ? 'success' : 'error'}
|
|
>
|
|
{inSyncReplicas && replicas && inSyncReplicas < replicas ? (
|
|
<Metrics.RedText>{inSyncReplicas}</Metrics.RedText>
|
|
) : (
|
|
inSyncReplicas
|
|
)}
|
|
<Metrics.LightText> of {replicas}</Metrics.LightText>
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Type">
|
|
<Tag color="gray">{internal ? 'Internal' : 'External'}</Tag>
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Segment Size" title="">
|
|
<BytesFormatted value={segmentSize} />
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Segment Count">
|
|
{segmentCount}
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Clean Up Policy">
|
|
<Tag color="gray">{cleanUpPolicy || 'Unknown'}</Tag>
|
|
</Metrics.Indicator>
|
|
<Metrics.Indicator label="Message Count">
|
|
{messageCount}
|
|
</Metrics.Indicator>
|
|
</Metrics.Section>
|
|
</Metrics.Wrapper>
|
|
<div>
|
|
<Table isFullwidth>
|
|
<thead>
|
|
<tr>
|
|
<TableHeaderCell title="Partition ID" />
|
|
<TableHeaderCell title="Replicas" />
|
|
<TableHeaderCell title="First Offset" />
|
|
<TableHeaderCell title="Next Offset" />
|
|
<TableHeaderCell title="Message Count" />
|
|
<TableHeaderCell title=" " />
|
|
</tr>
|
|
</thead>
|
|
<tbody>
|
|
{partitions?.map((partition: Partition) => (
|
|
<tr key={`partition-list-item-key-${partition.partition}`}>
|
|
<td>{partition.partition}</td>
|
|
<td>
|
|
{partition.replicas?.map((replica: Replica) => (
|
|
<ReplicaCell
|
|
leader={replica.leader}
|
|
key={`replica-list-item-key-${replica.broker}`}
|
|
>
|
|
{replica.broker}
|
|
</ReplicaCell>
|
|
))}
|
|
</td>
|
|
<td>{partition.offsetMin}</td>
|
|
<td>{partition.offsetMax}</td>
|
|
<td>{partition.offsetMax - partition.offsetMin}</td>
|
|
<td style={{ width: '5%' }}>
|
|
{!internal && !isReadOnly && cleanUpPolicy === 'DELETE' ? (
|
|
<Dropdown label={<VerticalElipsisIcon />} right>
|
|
<DropdownItem
|
|
onClick={() =>
|
|
clearTopicMessages({
|
|
clusterName,
|
|
topicName,
|
|
partitions: [partition.partition],
|
|
})
|
|
}
|
|
danger
|
|
>
|
|
Clear Messages
|
|
</DropdownItem>
|
|
</Dropdown>
|
|
) : null}
|
|
</td>
|
|
</tr>
|
|
))}
|
|
{partitions?.length === 0 && (
|
|
<tr>
|
|
<td colSpan={10}>No Partitions found</td>
|
|
</tr>
|
|
)}
|
|
</tbody>
|
|
</Table>
|
|
</div>
|
|
</>
|
|
);
|
|
};
|
|
|
|
export default Overview;
|